python烟花代码高级
时间: 2023-03-23 10:00:53 浏览: 455
以下是一个使用Python实现烟花效果的高级代码示例:
```python
import random
import math
import pygame
# 初始化 pygame 库
pygame.init()
# 设置窗口尺寸
width = 800
height = 600
screen = pygame.display.set_mode((width, height))
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义烟花类
class Firework:
def __init__(self, x, y):
self.x = x
self.y = y
self.color = (random.randint(50, 255), random.randint(50, 255), random.randint(50, 255))
self.radius = random.randint(5, 10)
self.vel = random.randint(1, 10)
def move(self):
self.y -= self.vel
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.radius)
# 定义爆炸效果类
class Explosion:
def __init__(self, x, y, color):
self.particles = []
self.color = color
for i in range(50):
speed = random.randint(1, 10)
angle = math.radians(random.randint(0, 360))
x_vel = speed * math.sin(angle)
y_vel = speed * math.cos(angle)
particle = [x, y, x_vel, y_vel, random.randint(1, 5)]
self.particles.append(particle)
def move(self):
for particle in self.particles:
particle[0] += particle[2]
particle[1] += particle[3]
particle[4] -= 0.1
particle[2] *= 0.95
particle[3] *= 0.95
def draw(self):
for particle in self.particles:
if particle[4] > 0:
pygame.draw.circle(screen, self.color, (int(particle[0]), int(particle[1])), int(particle[4]))
# 创建烟花列表
fireworks = []
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 添加新的烟花
if random.randint(0, 10) == 0:
fireworks.append(Firework(random.randint(0, width), height))
# 移动和绘制烟花
for firework in fireworks:
firework.move()
firework.draw()
# 烟花到达顶部,爆炸效果
if firework.y < 300:
fireworks.remove(firework)
explosion = Explosion(firework.x, firework.y, firework.color)
while len(fireworks) < 5:
fireworks.append(Firework(firework.x, firework.y))
# 移动和绘制爆炸效果
for firework in fireworks:
if firework.y < 300:
explosion.move()
explosion.draw()
# 更新屏幕
pygame.display.update
阅读全文
相关推荐
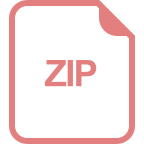
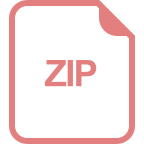
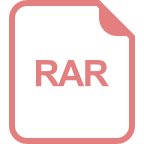



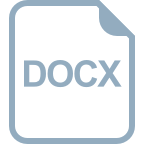
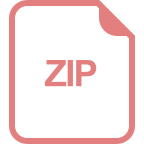



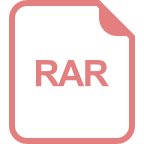
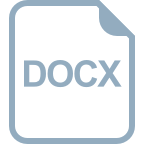
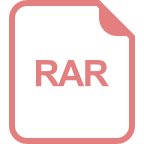
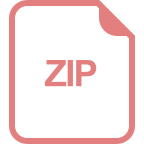
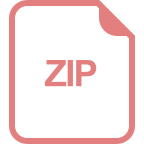
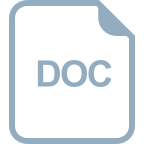
