opencv对图片中数字识别,并输出内容的代码
时间: 2024-05-04 07:22:15 浏览: 15
以下是一个基于OpenCV的简单数字识别的Python代码示例:
```python
import cv2
# 加载数字模板图像
template_images = []
for i in range(10):
template_images.append(cv2.imread(f"template_images/{i}.jpg", 0))
# 加载待识别的图片
image = cv2.imread("test_image.jpg")
# 转为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 应用高斯模糊
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# 进行二值化处理
_, thresh = cv2.threshold(blurred, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 查找轮廓
_, contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓
for contour in contours:
# 计算轮廓的外接矩形
(x, y, w, h) = cv2.boundingRect(contour)
# 如果外接矩形面积过小,则忽略该轮廓
if cv2.contourArea(contour) < 100:
continue
# 提取数字区域并缩放到模板图像大小
roi = cv2.resize(thresh[y:y + h, x:x + w], (20, 20), interpolation=cv2.INTER_AREA)
# 计算数字匹配分数
scores = []
for template in template_images:
result = cv2.matchTemplate(roi, template, cv2.TM_CCOEFF)
(_, score, _, _) = cv2.minMaxLoc(result)
scores.append(score)
# 输出匹配最高的数字
digit = scores.index(max(scores))
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(image, str(digit), (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 1.2, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Result", image)
cv2.waitKey(0)
```
代码中使用了一个包含0-9数字模板图像的文件夹`template_images`,这些图像应该是灰度图像,并且大小应该与待识别数字区域的大小相同。程序首先将待识别图像转为灰度图像并应用高斯模糊进行平滑处理,然后进行二值化处理并查找数字轮廓。遍历轮廓时,程序计算每个数字区域与数字模板图像的匹配分数,并输出匹配最高的数字。最后,程序将识别结果在原图像上标记并显示出来。
相关推荐
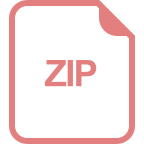
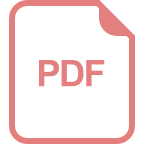
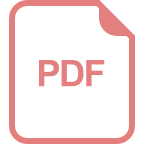














