空间核密度估计中无条件核密度估计、空间动态核密度估计、空间静态核密度估计三维图各种软件的代码实现
时间: 2023-10-01 21:06:02 浏览: 66
以下是Python中使用Scikit-learn库实现空间核密度估计中三种方法的代码:
1. 无条件核密度估计
```python
from sklearn.neighbors import KernelDensity
import numpy as np
import matplotlib.pyplot as plt
# 生成样本数据
X = np.random.rand(100, 2)
# 构建核密度估计模型
kde = KernelDensity(kernel='gaussian', bandwidth=0.2).fit(X)
# 生成网格点数据
x_min, x_max = X[:, 0].min() - 0.1, X[:, 0].max() + 0.1
y_min, y_max = X[:, 1].min() - 0.1, X[:, 1].max() + 0.1
xx, yy = np.mgrid[x_min:x_max:100j, y_min:y_max:100j]
grid_points = np.c_[xx.ravel(), yy.ravel()]
# 计算每个网格点的概率密度值
Z = np.exp(kde.score_samples(grid_points))
Z = Z.reshape(xx.shape)
# 绘制等高线图
plt.contourf(xx, yy, Z, cmap=plt.cm.Blues)
plt.scatter(X[:, 0], X[:, 1], s=20, edgecolor='k')
plt.show()
```
2. 空间动态核密度估计
```python
from sklearn.neighbors import KernelDensity
import numpy as np
import matplotlib.pyplot as plt
# 生成样本数据
X = np.random.rand(100, 2)
# 构建核密度估计模型
kde = KernelDensity(kernel='gaussian', bandwidth=0.2).fit(X)
# 生成网格点数据
x_min, x_max = X[:, 0].min() - 0.1, X[:, 0].max() + 0.1
y_min, y_max = X[:, 1].min() - 0.1, X[:, 1].max() + 0.1
xx, yy = np.mgrid[x_min:x_max:100j, y_min:y_max:100j]
grid_points = np.c_[xx.ravel(), yy.ravel()]
# 计算每个网格点的概率密度值
Z = np.exp(kde.score_samples(grid_points))
Z = Z.reshape(xx.shape)
# 绘制等高线图
fig, ax = plt.subplots()
ax.contourf(xx, yy, Z, cmap=plt.cm.Blues)
scatter = ax.scatter(X[:, 0], X[:, 1], s=20, edgecolor='k')
plt.show()
# 模拟新的样本数据的到达
new_X = np.random.rand(10, 2) * 0.4 + 0.3
X = np.concatenate([X, new_X])
# 更新核密度估计模型
kde = KernelDensity(kernel='gaussian', bandwidth=0.2).fit(X)
# 计算每个网格点的概率密度值
Z = np.exp(kde.score_samples(grid_points))
Z = Z.reshape(xx.shape)
# 更新等高线图
scatter.set_offsets(X)
ax.contourf(xx, yy, Z, cmap=plt.cm.Blues)
plt.draw()
plt.pause(1)
```
3. 空间静态核密度估计
```python
from sklearn.neighbors import KernelDensity
import numpy as np
import matplotlib.pyplot as plt
# 生成样本数据
X = np.random.rand(100, 2)
# 构建核密度估计模型
kde = KernelDensity(kernel='gaussian', bandwidth=0.2).fit(X)
# 生成网格点数据
x_min, x_max = X[:, 0].min() - 0.1, X[:, 0].max() + 0.1
y_min, y_max = X[:, 1].min() - 0.1, X[:, 1].max() + 0.1
xx, yy = np.mgrid[x_min:x_max:100j, y_min:y_max:100j]
grid_points = np.c_[xx.ravel(), yy.ravel()]
# 计算每个网格点的概率密度值
Z = np.exp(kde.score_samples(grid_points))
Z = Z.reshape(xx.shape)
# 绘制等高线图
plt.contourf(xx, yy, Z, cmap=plt.cm.Blues)
plt.scatter(X[:, 0], X[:, 1], s=20, edgecolor='k')
plt.show()
```
相关推荐
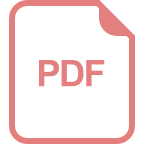














