c++项目怎么获取一个文件夹下所有的图片名
时间: 2023-08-31 12:06:54 浏览: 45
在 C++ 项目中,要获取一个文件夹下所有的图片文件名,您可以使用文件系统库来遍历目录并筛选出图片文件。以下是一个示例代码:
```cpp
#include <iostream>
#include <filesystem>
#include <vector>
#include <string>
namespace fs = std::filesystem;
std::vector<std::string> getAllImageFiles(const std::string& folderPath)
{
std::vector<std::string> imageFiles;
for (const auto& entry : fs::directory_iterator(folderPath))
{
if (entry.is_regular_file())
{
std::string extension = entry.path().extension().string();
if (extension == ".jpg" || extension == ".jpeg" || extension == ".png" || extension == ".bmp")
{
imageFiles.push_back(entry.path().filename().string());
}
}
}
return imageFiles;
}
int main()
{
std::string folderPath = "path/to/folder";
std::vector<std::string> imageFiles = getAllImageFiles(folderPath);
for (const auto& file : imageFiles)
{
std::cout << file << std::endl;
}
return 0;
}
```
在上述代码中,我们定义了一个函数 `getAllImageFiles`,该函数接受一个文件夹路径作为参数,并返回一个存储图片文件名的字符串向量。函数通过遍历目录中的每个文件,并检查文件的扩展名来筛选出图片文件。在这个例子中,我们只考虑了常见的图片格式(.jpg、.jpeg、.png、.bmp),您可以根据需要添加或修改扩展名的判断条件。
在 `main` 函数中,我们调用 `getAllImageFiles` 函数来获取文件夹中的所有图片文件名,并将其打印到标准输出。
请确保在编译和运行代码之前,将示例中的 `"path/to/folder"` 替换为实际的文件夹路径。另外,这是一个基于 C++17 的示例,您需要在编译时启用对应的语言标准。
相关推荐
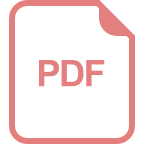
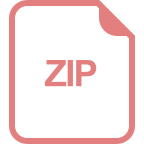














