s=np.random.randint(0, 100, size=5) print(s)
时间: 2023-11-13 13:06:17 浏览: 48
这段代码使用了 NumPy 库中的 `random` 函数生成了一个长度为 5、取值在 0~99 之间的随机整数数组。代码执行后会输出该数组。可以运行以下代码查看结果:
```python
import numpy as np
s = np.random.randint(0, 100, size=5)
print(s)
```
输出结果类似于:
```
[45 2 89 91 8]
```
相关问题
x1 = np.random.randint
根据提供的引用内容,没有找到与np.random.randint()相关的信息。但是,可以根据提供的信息介绍如何使用np.random生成随机整数数组。
要生成随机整数数组,可以使用np.random.randint()函数。该函数的语法如下:
```python
numpy.random.randint(low, high=None, size=None, dtype='l')
```
其中,参数说明如下:
- low:生成的随机数范围的下界(包含)。
- high:生成的随机数范围的上界(不包含)。如果未指定,则生成的随机数范围为[0, low)。
- size:生成的随机数数组的形状。可以是一个整数,表示生成一维数组的长度;也可以是一个元组,表示生成多维数组的形状。
- dtype:生成的随机数的数据类型。默认为int64。
下面是一个生成随机整数数组的例子:
```python
import numpy as np
# 生成一个长度为5,随机整数范围为[0, 10)的一维数组
x = np.random.randint(0, 10, 5)
print(x) # 输出:[7 9 3 5 2]
# 生成一个形状为(2, 3),随机整数范围为[0, 5)的二维数组
y = np.random.randint(0, 5, (2, 3))
print(y) # 输出:[[1 4 0]
# [3 2 1]]
```
a = np.random.randint
这行代码的意思是使用NumPy库中的random模块生成一个随机整数数组a,其中randint函数用于生成指定范围内的随机整数。具体来说,np.random.randint(low, high=None, size=None, dtype='l')函数的参数含义如下:
- low:生成的随机整数最小值(包含)。
- high:生成的随机整数最大值(不包含),如果不指定,则生成的随机整数范围为[0, low)。
- size:生成的随机整数数组的形状,可以是一个整数,表示生成一维数组,也可以是一个元组,表示生成多维数组。
- dtype:生成的随机整数的数据类型,默认为int64。
例如,如果想要生成一个形状为(3, 4)、元素值在[0, 10)之间的随机整数数组,可以使用以下代码:
```
import numpy as np
a = np.random.randint(0, 10, size=(3, 4))
print(a)
```
输出结果可能为:
```
[[5 9 2 7]
[6 8 7 7]
[7 7 8 1]]
```
相关推荐
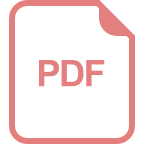
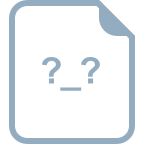
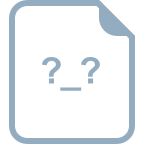












