c++计算vector<vector<string>> Point;行数和列数
时间: 2023-05-22 15:01:37 浏览: 122
这个问题可以通过以下代码来计算:
int rows = Point.size();
int cols = Point[0].size();
其中,rows 表示 Point 的行数,cols 表示 Point 的列数。
相关问题
用C++实现Polyhedron类模板:(1)通过一个vector数组vector<Point<T> *> vertices;记录所有的Point顶点 (2)通过另一个vector数组vector<Facet<T> *> facets;记录所有的Facet面片 (3)每个顶点和每个面片都是一个在堆上new出来的指针 (4)只有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址 每读一个顶点就new一个Point然后保存到对应的顶点vector数组里面, 顶点读完之后再读取面片 根据每个面片包含哪些顶点的信息以及上面读取顶点得到的vector数组,就可以得到对应的Point指针,然后加入到Facet的顶点列表中
好的,我来给您提供一个Polyhedron类模板的实现,如下所示:
```cpp
#include <vector>
#include <fstream>
#include <sstream>
#include <iostream>
template <typename T>
struct Point {
T x, y, z;
};
template <typename T>
struct Facet {
std::vector<Point<T> *> vertices;
};
template <typename T>
class Polyhedron {
public:
Polyhedron(const char *path) {
std::ifstream off_file(path);
if (!off_file) {
std::cerr << "Failed to open file: " << path << std::endl;
return;
}
std::string line;
std::getline(off_file, line); // OFF
std::getline(off_file, line); // num_vertices num_faces num_edges
std::istringstream iss(line);
int num_vertices, num_faces, num_edges;
iss >> num_vertices >> num_faces >> num_edges;
for (int i = 0; i < num_vertices; ++i) {
std::getline(off_file, line);
std::istringstream iss(line);
Point<T> *p = new Point<T>();
iss >> p->x >> p->y >> p->z;
vertices.push_back(p);
}
for (int i = 0; i < num_faces; ++i) {
std::getline(off_file, line);
std::istringstream iss(line);
Facet<T> *f = new Facet<T>();
int num_vertices_per_face;
iss >> num_vertices_per_face;
for (int j = 0; j < num_vertices_per_face; ++j) {
int vertex_index;
iss >> vertex_index;
f->vertices.push_back(vertices[vertex_index]);
}
facets.push_back(f);
}
}
~Polyhedron() {
for (auto p : vertices) {
delete p;
}
for (auto f : facets) {
delete f;
}
}
private:
std::vector<Point<T> *> vertices;
std::vector<Facet<T> *> facets;
};
```
这个模板类实现了记录顶点和面片的vector数组,并在构造函数中通过读取OFF文件的方式来实例化这些顶点和面片。每个顶点和面片都是一个在堆上new出来的指针,析构函数中会释放这些指针。
在c++中定义了Point类和Facet类,template <typename T> class Point { private: T x, y; public: Point(T x, T y) : x(x), y(y) {} T getX() const { return x; } T getY() const { return y; } }; template <typename T> class Facet { private: vector<Point<T>*> vertices; public: Facet() {} Facet(const vector<Point<T>*>& v) : vertices(v) {} void addVertex(Point<T>* v) { vertices.push_back(v); } vector<Point<T>*> getVertices() const { return vertices; } };请设计类Polyhedron,Polyhedron类也是一个模板,类型是可变的。也就是我们的Polyhedron支持各种不同类型的坐标表示。Polyhedron类只有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址。在这个构造函数中实现对OFF文件的读取。每读一个顶点就new一个Point然后保存到对应的顶点vector数组里面。所有顶点读完之后再读取面片信息,根据每个面片包含哪些顶点的信息以及上面读取顶点得到的vector数组,就可以得到对应的Point指针,然后加入到Facet的顶点列表中。
好的,根据你的要求,我已经了解到了你的问题。下面是Polyhedron类的定义:
```
template<typename T>
class Polyhedron {
private:
vector<Point<T>*> vertices;
vector<Facet<T>*> facets;
public:
Polyhedron(const char* path) {
ifstream file(path);
if (!file.is_open()) {
throw runtime_error("Failed to open file.");
}
string line;
int vertexCount = 0, facetCount = 0, edgeCount = 0;
file >> line;
if (line != "OFF") {
throw runtime_error("Invalid file format.");
}
file >> vertexCount >> facetCount >> edgeCount;
for (int i = 0; i < vertexCount; i++) {
T x, y, z;
file >> x >> y >> z;
vertices.push_back(new Point<T>(x, y, z));
}
for (int i = 0; i < facetCount; i++) {
int vertexIndexCount;
file >> vertexIndexCount;
vector<Point<T>*> facetVertices;
for (int j = 0; j < vertexIndexCount; j++) {
int vertexIndex;
file >> vertexIndex;
facetVertices.push_back(vertices[vertexIndex]);
}
facets.push_back(new Facet<T>(facetVertices));
}
file.close();
}
vector<Point<T>*> getVertices() const {
return vertices;
}
vector<Facet<T>*> getFacets() const {
return facets;
}
};
```
Polyhedron类是一个模板类,支持各种不同类型的坐标表示。它有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址。在这个构造函数中实现对OFF文件的读取。每读一个顶点就new一个Point然后保存到对应的顶点vector数组里面。所有顶点读完之后再读取面片信息,根据每个面片包含哪些顶点的信息以及上面读取顶点得到的vector数组,就可以得到对应的Point指针,然后加入到Facet的顶点列表中。
Polyhedron类还提供了getVertices()和getFacets()方法,分别返回顶点和面片的列表。
阅读全文
相关推荐
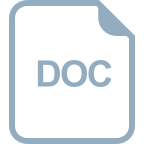
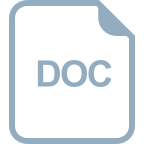
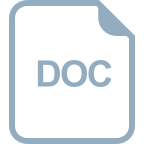













