请写一个程序 Polyhedron类只有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址。在这个构造函数中实现对OFF文件的读取。每读一个顶点就new一个Point然后保存到对应的顶点vector数组里面。所有顶点读完之后再读取面片信息,根据每个面片包含哪些顶点的信息以及上面读取顶点得到的vector数组,就可以得到对应的Point指针,然后加入到Facet的顶点列表中。Polyhedron类通过一个vector数组vector<Point<T> *> vertices;记录所有的Point顶点,通过另一个vector数组vector<Facet<T> *> facets;记录所有的Facet面片,每个顶点和每个面片都是一个在堆上new出来的指针。定义Point<T> get_low()函数来获得所有顶点坐标的下界。Point<T> get_high()函数来获得所有顶点坐标的上界。
时间: 2024-02-11 12:06:20 浏览: 121
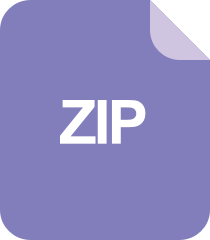
Polyhedron:多面体引擎 - 从 Tesseract 分叉
以下是一个可能的实现:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <sstream>
template <typename T>
struct Point {
T x, y, z;
Point(T x_, T y_, T z_) : x(x_), y(y_), z(z_) {}
};
template <typename T>
struct Facet {
std::vector<Point<T> *> vertices;
};
template <typename T>
class Polyhedron {
public:
Polyhedron(const char *path) {
std::ifstream ifs(path);
if (!ifs) {
std::cerr << "Failed to open file: " << path << std::endl;
return;
}
// 读取文件头
std::string line;
std::getline(ifs, line);
if (line != "OFF") {
std::cerr << "Invalid file format" << std::endl;
return;
}
// 读取顶点数和面片数
int num_vertices, num_facets, num_edges;
std::getline(ifs, line);
std::istringstream iss1(line);
iss1 >> num_vertices >> num_facets >> num_edges;
// 读取顶点
for (int i = 0; i < num_vertices; ++i) {
T x, y, z;
std::getline(ifs, line);
std::istringstream iss2(line);
iss2 >> x >> y >> z;
Point<T> *p = new Point<T>(x, y, z);
vertices.push_back(p);
}
// 读取面片
for (int i = 0; i < num_facets; ++i) {
int num_vertices_per_facet;
std::getline(ifs, line);
std::istringstream iss3(line);
iss3 >> num_vertices_per_facet;
Facet<T> *f = new Facet<T>;
for (int j = 0; j < num_vertices_per_facet; ++j) {
int index;
iss3 >> index;
f->vertices.push_back(vertices[index]);
}
facets.push_back(f);
}
}
~Polyhedron() {
for (auto p : vertices)
delete p;
for (auto f : facets)
delete f;
}
Point<T> get_low() const {
T x_min = vertices[0]->x, y_min = vertices[0]->y, z_min = vertices[0]->z;
for (auto p : vertices) {
if (p->x < x_min)
x_min = p->x;
if (p->y < y_min)
y_min = p->y;
if (p->z < z_min)
z_min = p->z;
}
return Point<T>(x_min, y_min, z_min);
}
Point<T> get_high() const {
T x_max = vertices[0]->x, y_max = vertices[0]->y, z_max = vertices[0]->z;
for (auto p : vertices) {
if (p->x > x_max)
x_max = p->x;
if (p->y > y_max)
y_max = p->y;
if (p->z > z_max)
z_max = p->z;
}
return Point<T>(x_max, y_max, z_max);
}
private:
std::vector<Point<T> *> vertices;
std::vector<Facet<T> *> facets;
};
int main() {
Polyhedron<double> polyhedron("example.off");
Point<double> low = polyhedron.get_low();
Point<double> high = polyhedron.get_high();
std::cout << "Low: " << low.x << " " << low.y << " " << low.z << std::endl;
std::cout << "High: " << high.x << " " << high.y << " " << high.z << std::endl;
return 0;
}
```
需要注意的是,这里只是一个简单的实现,还有很多细节需要处理,例如文件格式的检查、错误处理、内存管理等问题,需要根据具体需求进行完善。
阅读全文
相关推荐
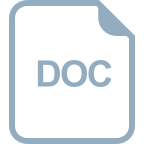
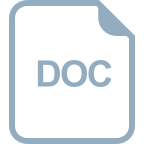







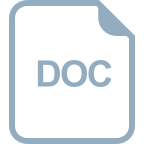
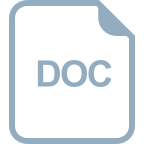
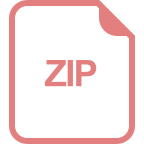
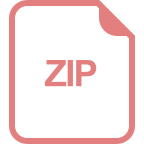