python做一个飞机小游戏
时间: 2023-10-08 07:14:21 浏览: 57
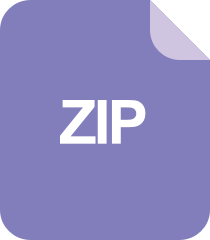
Python实现的飞机小游戏
以下是一个简单的飞机小游戏的Python代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口的大小和标题
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("飞机大战")
# 加载游戏资源
background_image = pygame.image.load("background.png").convert()
player_image = pygame.image.load("player.png").convert_alpha()
enemy_image = pygame.image.load("enemy.png").convert_alpha()
bullet_image = pygame.image.load("bullet.png").convert_alpha()
# 设置游戏音效
bullet_sound = pygame.mixer.Sound("bullet.wav")
explosion_sound = pygame.mixer.Sound("explosion.wav")
# 定义游戏对象
class GameObject:
def __init__(self, x, y, image):
self.x = x
self.y = y
self.image = image
self.width = image.get_width()
self.height = image.get_height()
def draw(self, surface):
surface.blit(self.image, (self.x, self.y))
def collide(self, other):
if self.x + self.width > other.x and self.x < other.x + other.width and \
self.y + self.height > other.y and self.y < other.y + other.height:
return True
else:
return False
# 定义玩家飞机对象
class Player(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = 5
self.bullets = []
self.score = 0
def move_left(self):
self.x -= self.speed
if self.x < 0:
self.x = 0
def move_right(self):
self.x += self.speed
if self.x > screen_width - self.width:
self.x = screen_width - self.width
def fire(self):
bullet = Bullet(self.x + self.width // 2 - bullet_image.get_width() // 2, self.y, bullet_image)
self.bullets.append(bullet)
bullet_sound.play()
# 定义敌机对象
class Enemy(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = 3
def move(self):
self.y += self.speed
# 定义子弹对象
class Bullet(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = 10
def move(self):
self.y -= self.speed
# 创建玩家飞机对象
player = Player(screen_width // 2 - player_image.get_width() // 2, screen_height - player_image.get_height() - 10, player_image)
# 创建敌机列表
enemies = []
# 设置游戏循环
running = True
while running:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
player.move_left()
elif event.key == pygame.K_RIGHT:
player.move_right()
elif event.key == pygame.K_SPACE:
player.fire()
# 更新游戏状态
for enemy in enemies:
enemy.move()
if enemy.collide(player):
explosion_sound.play()
running = False
for bullet in player.bullets:
if bullet.collide(enemy):
explosion_sound.play()
player.score += 10
player.bullets.remove(bullet)
enemies.remove(enemy)
if enemy.y > screen_height:
enemies.remove(enemy)
for bullet in player.bullets:
bullet.move()
if bullet.y < 0:
player.bullets.remove(bullet)
if len(enemies) < 10:
enemy = Enemy(random.randint(0, screen_width - enemy_image.get_width()), -enemy_image.get_height(), enemy_image)
enemies.append(enemy)
# 绘制游戏界面
screen.blit(background_image, (0, 0))
player.draw(screen)
for enemy in enemies:
enemy.draw(screen)
for bullet in player.bullets:
bullet.draw(screen)
score_text = pygame.font.SysFont(None, 36).render("Score: " + str(player.score), True, (255, 255, 255))
screen.blit(score_text, (10, 10))
pygame.display.update()
# 结束游戏
pygame.quit()
```
游戏玩法:
玩家使用左右箭头控制飞机移动,空格键发射子弹。敌机会从上往下移动,玩家需要尽可能地击落敌机并躲避敌机的攻击。游戏结束条件为:玩家被敌机撞击。游戏得分规则为:每击落一个敌机得10分。
阅读全文
相关推荐
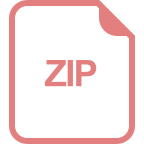











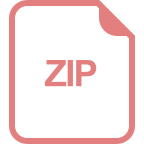
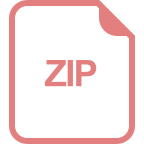