用Python写一个根据一个循环体进行计时的代码
时间: 2023-05-23 16:02:28 浏览: 52
可以使用Python的time和datetime模块来编写一个根据循环体进行计时的代码,在循环体的开始和结束时间记录下来之后,可以计算出总共循环的时间。下面是一个示例代码:
```python
import time
start_time = time.time()
for i in range(10):
print(i)
time.sleep(1)
end_time = time.time()
total_time = end_time - start_time
print("循环花费的总时间为:", total_time)
```
这段代码会循环输出数字并且让程序暂停1秒钟,然后记录下循环开始和结束的时间,最后计算出循环花费的总时间。
相关问题
用Python写一个根据一个循环体进行计时的代码,并通过界面显示进度条
当然可以!以下是一个示例代码:
```
import time
import sys
# 设置循环次数
total = 10
# 进度条宽度
bar_length = 20
for i in range(total):
# 打印进度条
percent = i / (total-1)
bar = '#' * int(percent * bar_length)
space = ' ' * (bar_length - len(bar))
sys.stdout.write('\r正在执行(%d/%d):%s |%s| %d%% ' % (i+1, total, bar+space, int(percent*100)))
sys.stdout.flush()
# 让程序等待 1 秒钟
time.sleep(1)
```
这个代码可以根据给定的循环次数,在命令行界面(也可以用 PyQT 或 TKinter 等图形界面库)中显示一个进度条,并在循环中执行其他操作。每次循环结束后,进度条会更新,并显示进度百分比。
帮我用python写一个俄罗斯方块游戏的代码。
好的,我可以回答这个问题。以下是一个简单的俄罗斯方块游戏的 Python 代码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 游戏窗口大小
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 600
# 方块大小
BLOCK_SIZE = 20
# 方块的形状
SHAPES = [
[[1, 1, 1], [, 1, ]],
[[2, 2], [2, 2]],
[[3, 3, ], [, 3, 3]],
[[, 4, 4], [4, 4, ]],
[[5, 5, 5, 5]],
[[6, 6, 6], [, , 6]],
[[7, 7, 7], [7, , ]],
]
# 方块的颜色
COLORS = [
(, , ),
(255, , ),
(, 255, ),
(, , 255),
(255, 255, ),
(255, , 255),
(, 255, 255),
]
# 创建游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("俄罗斯方块")
# 创建字体对象
font = pygame.font.Font(None, 36)
# 创建一个计时器
clock = pygame.time.Clock()
# 定义一个方块类
class Block:
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
self.color = random.randint(1, len(COLORS) - 1)
self.rotation =
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.shape)
def get_current_shape(self):
return self.shape[self.rotation]
def draw(self):
current_shape = self.get_current_shape()
for i in range(len(current_shape)):
for j in range(len(current_shape[i])):
if current_shape[i][j] != :
pygame.draw.rect(window, COLORS[self.color], (self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
def move_down(self):
self.y += BLOCK_SIZE
def move_left(self):
self.x -= BLOCK_SIZE
def move_right(self):
self.x += BLOCK_SIZE
# 定义一个游戏类
class Game:
def __init__(self):
self.score =
self.level = 1
self.lines =
self.block = None
self.next_block = Block(WINDOW_WIDTH / 2 - BLOCK_SIZE * 2, , SHAPES[random.randint(, len(SHAPES) - 1)])
self.is_game_over = False
self.grid = [[ for _ in range(WINDOW_WIDTH // BLOCK_SIZE)] for _ in range(WINDOW_HEIGHT // BLOCK_SIZE)]
def new_block(self):
self.block = self.next_block
self.next_block = Block(WINDOW_WIDTH / 2 - BLOCK_SIZE * 2, , SHAPES[random.randint(, len(SHAPES) - 1)])
if self.check_collision(self.block):
self.is_game_over = True
def check_collision(self, block):
current_shape = block.get_current_shape()
for i in range(len(current_shape)):
for j in range(len(current_shape[i])):
if current_shape[i][j] != :
x = block.x + j * BLOCK_SIZE
y = block.y + i * BLOCK_SIZE
if x < or x >= WINDOW_WIDTH or y >= WINDOW_HEIGHT or self.grid[y // BLOCK_SIZE][x // BLOCK_SIZE] != :
return True
return False
def add_to_grid(self, block):
current_shape = block.get_current_shape()
for i in range(len(current_shape)):
for j in range(len(current_shape[i])):
if current_shape[i][j] != :
x = block.x + j * BLOCK_SIZE
y = block.y + i * BLOCK_SIZE
self.grid[y // BLOCK_SIZE][x // BLOCK_SIZE] = block.color
def check_lines(self):
lines_cleared =
for i in range(len(self.grid)):
if all(self.grid[i]):
self.grid.pop(i)
self.grid.insert(, [ for _ in range(WINDOW_WIDTH // BLOCK_SIZE)])
lines_cleared += 1
self.lines += lines_cleared
self.score += lines_cleared ** 2 * 100
self.level = 1 + self.lines // 10
def draw_grid(self):
for i in range(len(self.grid)):
for j in range(len(self.grid[i])):
pygame.draw.rect(window, COLORS[self.grid[i][j]], (j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 1)
def draw_score(self):
score_text = font.render("Score: " + str(self.score), True, (255, 255, 255))
level_text = font.render("Level: " + str(self.level), True, (255, 255, 255))
lines_text = font.render("Lines: " + str(self.lines), True, (255, 255, 255))
window.blit(score_text, (10, 10))
window.blit(level_text, (10, 50))
window.blit(lines_text, (10, 90))
def draw_game_over(self):
game_over_text = font.render("Game Over", True, (255, , ))
window.blit(game_over_text, (WINDOW_WIDTH / 2 - game_over_text.get_width() / 2, WINDOW_HEIGHT / 2 - game_over_text.get_height() / 2))
def update(self):
if not self.is_game_over:
if self.block is None:
self.new_block()
else:
self.block.move_down()
if self.check_collision(self.block):
self.block.move_up()
self.add_to_grid(self.block)
self.check_lines()
self.block = None
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT and self.block is not None:
self.block.move_left()
if self.check_collision(self.block):
self.block.move_right()
elif event.key == pygame.K_RIGHT and self.block is not None:
self.block.move_right()
if self.check_collision(self.block):
self.block.move_left()
elif event.key == pygame.K_UP and self.block is not None:
self.block.rotate()
if self.check_collision(self.block):
self.block.rotate()
self.block.rotate()
self.block.rotate()
elif event.key == pygame.K_DOWN and self.block is not None:
self.block.move_down()
if self.check_collision(self.block):
self.block.move_up()
self.add_to_grid(self.block)
self.check_lines()
self.block = None
# 绘制游戏界面
window.fill((, , ))
self.draw_grid()
if self.block is not None:
self.block.draw()
self.next_block.draw()
self.draw_score()
if self.is_game_over:
self.draw_game_over()
# 更新窗口
pygame.display.update()
# 控制游戏帧率
clock.tick(30)
# 创建一个游戏对象
game = Game()
# 游戏循环
while True:
game.update()
```
希望这个代码对你有所帮助!
相关推荐
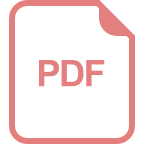
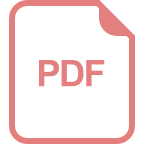












