c++ thread join函数的用法
时间: 2023-05-23 07:07:16 浏览: 64
A:c语言中,pthread_join函数的功能是等待一个线程结束。thread join函数的用法如下:
```
#include <pthread.h>
int pthread_join(pthread_t thread, void **retval);
```
- thread:需要等待结束的线程的标识符。
- retval:指向线程退出状态的指针(该指针指向的对象在线程退出时被传递给pthread_exit()函数)。
pthread_join()函数会一直阻塞当前线程,直到指定的线程结束后才返回,或者如果指定的线程已经结束,则立即返回。当pthread_join()函数返回时,指定线程的资源已经被回收。如果pthread_join()函数返回0,则表示成功等待指定线程结束;如果返回一个非零值,则表示出现了错误。
在线程函数中最后使用pthread_exit()函数,是为了让线程本身能够返回一个状态值,这个值会被传递给参数retval。如果没有传递参数,可以将它设为NULL。线程函数中的pthread_exit()函数不是必须的。如果不调用该函数,线程也会在函数返回时自动退出。
相关问题
c++ thread join 源码
c语言中的pthread_join函数是pthread库提供的一个函数。该函数的作用是等待指定线程执行结束。在使用pthread库编写多线程程序时,在主线程中使用pthread_join函数可以确保所有其他线程都已经退出,以便正确地终止程序的执行。
pthread_join函数的方法声明如下:
``` C
int pthread_join(pthread_t thread, void **retval);
```
其中,thread是传递给pthread_create函数创建的线程标识符,retval是指向存储线程返回值的指针。
pthread_join函数的源码实现比较简单。主要的实现过程是利用轮询来等待线程的终止。具体代码如下:
``` C
int pthread_join(pthread_t thread, void **retval)
{
thread_t *t = (thread_t *) thread;
spin_lock(&t->lock);
while (t->state == THREAD_STATE_RUNNING) {
spin_unlock(&t->lock);
yield(); //让出CPU
spin_lock(&t->lock);
}
spin_unlock(&t->lock);
/* 返回线程退出码 */
if (retval) {
*retval = t->ret;
}
return 0;
}
```
在代码中,首先通过pthread_t类型转换成thread_t标识符。借助t->state实现了线程的轮询等待,如果线程的状态为THREAD_STATE_RUNNING,主线程就会让出CPU,执行其他线程,直到等待线程的状态变为非运行状态。最后,通过指针传递的方式将线程的返回值赋给retval指针,并返回0。
C++ thread函数
在C++中,std::thread类用于创建和管理线程。它有多个构造函数,其中一些常用的构造函数有以下几种:
1. thread():默认构造函数,创建一个空的线程对象。
2. thread(thread&& other):移动构造函数,用于将一个线程对象的所有权转移到另一个线程对象。
3. template<class Function, class... Args> explicit thread(Function&& f, Args&&... args):带有函数和参数的构造函数,用于创建一个新线程并执行指定的函数。
4. thread(const thread&)=delete:禁用的拷贝构造函数,确保线程对象不能被复制。
以下是一个使用std::thread创建线程的简单例子,其中调用thread对象的构造函数来执行指定的函数,并通过join()函数等待线程执行完成:
```cpp
#include <iostream>
#include <thread>
void myfunc() {
std::cout << "myfunc\n";
// do something ...
}
int main() {
std::thread t1(myfunc);
t1.join();
return 0;
}
```
如果想要使用std::thread创建多个线程,可以将thread对象存储在vector容器中,并使用循环来创建和加入线程:
```cpp
#include <iostream>
#include <thread>
#include <vector>
void foo(int n) {
std::cout << "foo() " << n << std::endl;
}
int main() {
std::vector<std::thread> threads;
for (int i = 0; i < 3; i++) {
threads.push_back(std::thread(foo, i));
}
for (int i = 0; i < 3; i++) {
threads[i].join();
}
std::cout << "main() exit.\n";
return 0;
}
```
在参数传递方面,可以使用传引用的方式将参数传递给线程函数。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [C++ thread用法总结(整理)](https://blog.csdn.net/fuhanghang/article/details/114818215)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [C++中的thread最全用法归纳](https://blog.csdn.net/zzh516451964zzh/article/details/128421574)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
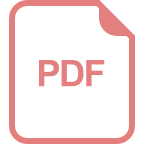
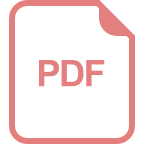
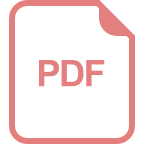












