c++语言分别给出如下5个文件:student.h、student.cpp、teacher.h、teacher.cpp和 main.cpp。在正确的位置,添加编译预处理命令;并将它们组织成一个工程, 编译执行。 b) 在Student类中,添加一个私有的静态数据成员count,用于统计学生总数。并 设计相应的静态成员函数showCount,以输出当前学生总数(注意:添加count 成员之后,要编写正确的复制构造函数和
时间: 2023-05-21 14:00:54 浏览: 144
赋值运算符重载函数,以确保count 成员的正确性)。以下是代码示例:
// student.h
#ifndef STUDENT_H
#define STUDENT_H
class Student {
public:
Student();
Student(const Student& other);
~Student();
Student& operator=(const Student& other);
void setName(const char* name);
const char* getName() const;
static void showCount();
private:
char* m_name;
static int s_count;
};
#endif // STUDENT_H
// student.cpp
#include "student.h"
int Student::s_count = 0;
Student::Student() : m_name(nullptr) {
++s_count;
}
Student::Student(const Student& other) : m_name(nullptr) {
setName(other.m_name);
++s_count;
}
Student::~Student() {
delete[] m_name;
--s_count;
}
Student& Student::operator=(const Student& other) {
if (this != &other) {
setName(other.m_name);
}
return *this;
}
void Student::setName(const char* name) {
delete[] m_name;
m_name = new char[strlen(name) + 1];
strcpy(m_name, name);
}
const char* Student::getName() const {
return m_name;
}
void Student::showCount() {
std::cout << "Total number of students: " << s_count << std::endl;
}
// teacher.h
#ifndef TEACHER_H
#define TEACHER_H
class Teacher {
public:
Teacher();
~Teacher();
void setName(const char* name);
const char* getName() const;
private:
char* m_name;
};
#endif // TEACHER_H
// teacher.cpp
#include "teacher.h"
Teacher::Teacher() : m_name(nullptr) {}
Teacher::~Teacher() {
delete[] m_name;
}
void Teacher::setName(const char* name) {
delete[] m_name;
m_name = new char[strlen(name) + 1];
strcpy(m_name, name);
}
const char* Teacher::getName() const {
return m_name;
}
// main.cpp
#include "student.h"
#include "teacher.h"
int main() {
Student s1;
s1.setName("Alice");
Student::showCount();
Student s2(s1);
s2.setName("Bob");
Student::showCount();
Student s3;
s3 = s2;
s3.setName("Charlie");
Student::showCount();
Teacher t1;
t1.setName("Mr. Smith");
std::cout << "Student 1: " << s1.getName() << std::endl;
std::cout << "Student 2: " << s2.getName() << std::endl;
std::cout << "Student 3: " << s3.getName() << std::endl;
std::cout << "Teacher: " << t1.getName() << std::endl;
return 0;
}
阅读全文
相关推荐
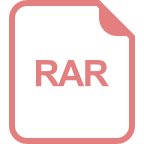
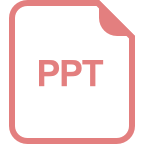

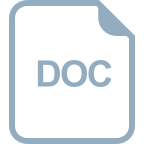













