模拟设计一个磁盘驱动调度程序,对磁盘进行移臂调度,观察驱动调度程序的动态运行过程,并输出移动臂的移动顺序和移动总柱面数。使用SSTF算法,C++语言 【说明和要求】1、假定某个磁盘组共有 200 个柱面,由外向里顺序编号(0—199); 2、设定磁头当前所在位置(柱面号)及移动方向,程序随机生成柱面访问请求序列(需 12 个以上); 3、程序界面上输入磁盘移臂调度算法序号并执行,输出移动臂的移动顺序和总柱面数等结 果
时间: 2024-02-24 22:58:47 浏览: 57
好的,我会尽力回答你的问题,但是我是一个自然语言生成AI模型,无法直接进行程序设计和运行。不过,我可以为你提供一个基本的磁盘调度程序实现思路:
1. 定义磁头当前所在位置和移动方向,以及生成柱面访问请求序列。
2. 使用SSTF算法对请求序列进行排序,选择最近的请求进行磁头移动。
3. 计算每次移动的柱面数,并将移动顺序记录下来。
4. 执行完所有请求后,输出移动顺序和移动总柱面数等结果。
以下是一个简单的C++语言实现示例:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <cmath>
using namespace std;
// 定义请求结构体
struct Request {
int cylinder; // 请求的柱面号
bool direction; // 请求的方向(1:向内,0:向外)
};
// SSTF算法
int sstf(vector<Request>& requests, int startCylinder, bool startDirection) {
int totalCylinder = 0; // 移动总柱面数
int currentCylinder = startCylinder; // 当前柱面
bool currentDirection = startDirection; // 当前移动方向(1:向内,0:向外)
vector<int> order; // 移动顺序
while (!requests.empty()) {
// 找出距离当前柱面最近的请求
int minDistance = abs(requests[0].cylinder - currentCylinder);
int minIndex = 0;
for (int i = 1; i < requests.size(); i++) {
int distance = abs(requests[i].cylinder - currentCylinder);
if (distance < minDistance) {
minDistance = distance;
minIndex = i;
}
}
// 计算移动距离和总柱面数
totalCylinder += minDistance;
order.push_back(requests[minIndex].cylinder);
// 更新当前柱面和方向
currentCylinder = requests[minIndex].cylinder;
currentDirection = (currentCylinder < currentDirection) ? 0 : 1;
// 删除已处理的请求
requests.erase(requests.begin() + minIndex);
}
// 输出移动顺序和移动总柱面数
cout << "Move order: ";
for (int i = 0; i < order.size(); i++) {
cout << order[i] << " ";
}
cout << endl;
cout << "Total cylinder: " << totalCylinder << endl;
return totalCylinder;
}
int main() {
// 定义请求序列
vector<Request> requests = {
{50, 1},
{95, 0},
{130, 1},
{10, 1},
{45, 0},
{180, 0},
{120, 1},
{75, 0},
{5, 1},
{170, 1},
{20, 0},
{100, 1}
};
// 随机生成请求序列
// ...
// 执行SSTF算法
int startCylinder = 100; // 起始柱面
bool startDirection = 1; // 起始方向(1:向内,0:向外)
int totalCylinder = sstf(requests, startCylinder, startDirection);
return 0;
}
```
注意,这只是一个简单的实现示例,实际的磁盘调度程序需要考虑更多的因素,如磁盘访问时间、多道批处理等。此外,程序中的请求序列可以通过随机数生成,或者从文件中读取。
相关推荐
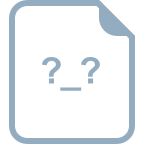
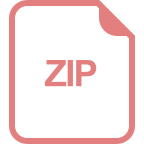















