为什么没有曲线 # 创建一个画布 fig, ax = plt.subplots() # 绘制连接散点图 plt.subplot(231), ax.plot(x, y1, 'r-', label='W') plt.subplot(232), ax.plot(x, y2, 'b-', label='i') plt.subplot(233), ax.plot(x, y3, 'g-', label='K') # 设置图像标题和轴标签 ax.set_title('Scatter Plot with Lines') ax.set_xlabel('X-axis') ax.set_ylabel('Y-axis') plt.show()
时间: 2023-08-18 09:06:11 浏览: 106
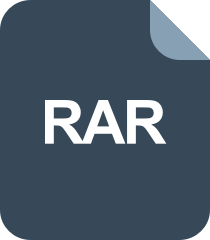
python 绘图---2D、3D散点图、折线图、曲面图_2D3D散点图折线图绘制_python_

这段代码中,绘制曲线的部分存在问题。 `plt.subplot(231)` 这样的语法是不正确的,因为 `plt.subplot()` 函数的返回值是 AxesSubplot 对象,而不是一个元组。因此,可以将绘图命令与 `plt.subplot()` 函数分开,并将其分配给一个变量,然后使用该变量来添加曲线。另外,这段代码中没有给数据 x 和 y1、y2、y3 赋值,所以绘图的数据不正确。下面是修改后的代码示例:
```
import matplotlib.pyplot as plt
import numpy as np
# 生成测试数据
x = np.arange(0, 10, 0.1)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
# 创建一个画布
fig, ax = plt.subplots()
# 绘制连接散点图
ax.plot(x, y1, 'r-', label='W')
ax.plot(x, y2, 'b-', label='i')
ax.plot(x, y3, 'g-', label='K')
# 设置图像标题和轴标签
ax.set_title('Scatter Plot with Lines')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
# 添加图例
ax.legend()
# 显示图像
plt.show()
```
这段代码将生成包含三条曲线的图形,并将其显示出来。
阅读全文
相关推荐
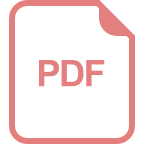
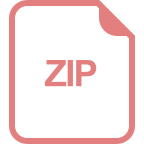















