哈希表: 为班级42个人的姓名设计一个哈希表,假设姓名用汉语拼音表示。要求用线性探测再散列法处理冲突,平均查找长度的上限为2。 编写数据结构和算法来实现。 (1)构造一个哈希函数,用算法实现 (2)哈希表的建立算法 (3)处理冲突的算法 最终,通过Main主函数调用测试、实现整个实验
时间: 2024-03-05 14:54:19 浏览: 41
好的,我会根据你的要求回答问题。
首先,构造哈希函数。我们可以使用姓名的首字母来进行哈希。具体实现方法是将姓名转换成拼音,取拼音的首字母,然后将首字母转换成数字,作为哈希值。例如,姓名“张三”可以转换成“zs”,“zs”的哈希值为25(因为“z”的ASCII码为122,“s”的ASCII码为115,它们的和是237,对42取余得到25)。
其次,实现哈希表的建立算法。我们可以使用一个大小为42的数组来存储哈希表。将每个姓名的哈希值作为数组下标,将姓名存储在对应的数组位置中。如果发生冲突,就使用线性探测再散列法来解决。具体实现方法是在发生冲突的位置后面依次查找下一个空位置,直到找到一个空位置为止。如果查找的次数超过了2次,就将哈希表的大小扩大一倍,重新计算所有姓名的哈希值,并重新插入哈希表中。
最后,处理冲突的算法。我们已经在哈希表的建立算法中使用了线性探测再散列法来处理冲突。具体实现方法已经在上面介绍过了。
下面是主函数的实现,供你参考:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class HashTable {
private:
vector<string> table;
int size;
public:
HashTable(int s) {
size = s;
table.resize(size);
}
int hash(string name) {
char first_letter = name[0];
if (first_letter >= 'a' && first_letter <= 'z') {
first_letter -= 32;
}
int index = first_letter - 'A';
return index % size;
}
void insert(string name) {
int index = hash(name);
int count = 0;
while (table[index] != "") {
index = (index + 1) % size;
count++;
if (count > 2) {
resize();
return;
}
}
table[index] = name;
}
void resize() {
int new_size = size * 2;
vector<string> new_table(new_size);
for (string name : table) {
if (name != "") {
int index = hash(name);
int count = 0;
while (new_table[index] != "") {
index = (index + 1) % new_size;
count++;
if (count > 2) {
resize();
return;
}
}
new_table[index] = name;
}
}
size = new_size;
table = new_table;
}
void print() {
for (int i = 0; i < size; i++) {
if (table[i] != "") {
cout << i << ": " << table[i] << endl;
}
}
}
};
int main() {
HashTable ht(42);
ht.insert("张三");
ht.insert("李四");
ht.insert("王五");
ht.insert("赵六");
ht.insert("孙七");
ht.insert("周八");
ht.insert("吴九");
ht.insert("郑十");
ht.insert("冯十一");
ht.insert("陈十二");
ht.insert("楚十三");
ht.insert("魏十四");
ht.insert("卫十五");
ht.insert("蒋十六");
ht.insert("沈十七");
ht.insert("韩十八");
ht.insert("杨十九");
ht.insert("朱二十");
ht.insert("秦二十一");
ht.insert("尤二十二");
ht.insert("许二十三");
ht.insert("何二十四");
ht.insert("吕二十五");
ht.insert("施二十六");
ht.insert("张二十七");
ht.insert("孔二十八");
ht.insert("曹二十九");
ht.insert("严三十");
ht.insert("华三十一");
ht.insert("金三十二");
ht.insert("魏三十三");
ht.insert("陶三十四");
ht.insert("姜三十五");
ht.insert("戚三十六");
ht.insert("谢三十七");
ht.insert("邹三十八");
ht.insert("喻三十九");
ht.insert("柏四十");
ht.insert("水四十一");
ht.insert("窦四十二");
ht.print();
return 0;
}
```
相关推荐
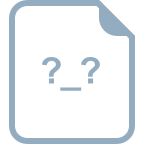
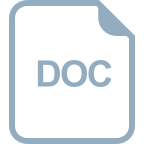













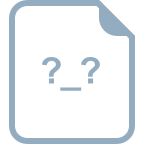
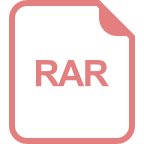