用python写一个网格交易策略代码
时间: 2023-12-17 20:44:30 浏览: 123
好的,以下是一个简单的Python代码实现网格交易策略的示例:
```python
import ccxt
import time
# 初始化交易所
exchange = ccxt.binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_SECRET_KEY',
'enableRateLimit': True
})
# 定义交易对和参数
symbol = 'BTC/USDT'
grid_size = 10 # 网格数量
grid_amount = 0.001 # 每格交易量
# 获取当前价格
ticker = exchange.fetch_ticker(symbol)
price = ticker['last']
# 计算网格价格
grid_prices = []
for i in range(grid_size):
grid_price = price * (1 - 0.02 * (i - grid_size // 2))
grid_prices.append(grid_price)
# 开始交易
while True:
# 获取当前价格
ticker = exchange.fetch_ticker(symbol)
price = ticker['last']
# 判断是否需要交易
if price <= grid_prices[0]:
# 买入
order = exchange.create_order(symbol, 'limit', 'buy', grid_amount, grid_prices[0])
print('Buy Order Created: ', order)
# 更新网格价格
grid_prices.pop(0)
grid_price = price * (1 + 0.02 * (grid_size // 2))
grid_prices.append(grid_price)
elif price >= grid_prices[-1]:
# 卖出
order = exchange.create_order(symbol, 'limit', 'sell', grid_amount, grid_prices[-1])
print('Sell Order Created: ', order)
# 更新网格价格
grid_prices.pop(-1)
grid_price = price * (1 - 0.02 * (grid_size // 2))
grid_prices.insert(0, grid_price)
# 休眠1分钟
time.sleep(60)
```
上述代码使用CCXT库连接Binance交易所,并使用BTC/USDT交易对实现网格交易策略。其中,`grid_size`表示网格数量,`grid_amount`表示每格交易量,代码中以0.001BTC为例。在运行代码前,需要将`YOUR_API_KEY`和`YOUR_SECRET_KEY`替换为您自己的API密钥。运行代码后,程序会自动进行网格交易,当价格达到网格价格时,程序会自动下单买入或卖出。
阅读全文
相关推荐
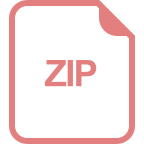
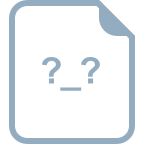


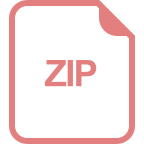
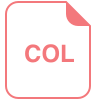
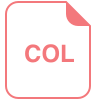










