c++ STL pair
时间: 2023-09-09 07:10:24 浏览: 60
STL pair 是 C++ STL(标准模板库)中的一个模板类,用于存储两个不同类型的数据。它的定义如下:
```
template <class T1, class T2>
struct pair {
typedef T1 first_type;
typedef T2 second_type;
T1 first;
T2 second;
// 构造函数
pair();
pair(const T1& x, const T2& y);
template<class U, class V> pair(const pair<U, V>& p);
// 操作符重载
pair& operator=(const pair& p);
template<class U, class V> pair& operator=(const pair<U, V>& p);
void swap(pair& p);
};
```
其中,`first_type` 和 `second_type` 分别表示 `pair` 的两个元素的类型。`first` 和 `second` 分别表示 `pair` 的两个元素的值。`pair` 的构造函数可以用来初始化两个元素的值,而操作符重载可以用来进行两个 `pair` 对象的比较和赋值。
下面是一个使用 `pair` 的例子:
```cpp
#include <iostream>
#include <utility>
using namespace std;
int main()
{
pair<string, int> p1("Hello", 123);
cout << "p1.first = " << p1.first << endl;
cout << "p1.second = " << p1.second << endl;
pair<string, int> p2 = make_pair("World", 456);
cout << "p2.first = " << p2.first << endl;
cout << "p2.second = " << p2.second << endl;
if (p1 < p2) {
cout << "p1 is less than p2" << endl;
} else {
cout << "p1 is greater than or equal to p2" << endl;
}
p1 = p2;
cout << "After assignment, p1.first = " << p1.first << endl;
cout << "After assignment, p1.second = " << p1.second << endl;
return 0;
}
```
输出结果为:
```
p1.first = Hello
p1.second = 123
p2.first = World
p2.second = 456
p1 is less than p2
After assignment, p1.first = World
After assignment, p1.second = 456
```
相关推荐
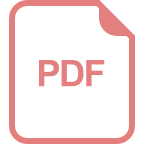
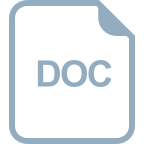
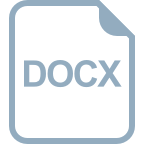














