def get_step(x, y, z): max_point = [x, y, z] print('the farthest node is:', max_point) ture_x, ture_y, ture_z = max_point[0], max_point[1], max_point[2] step_x, step_y, step_z = (max_point[0])/dis, (max_point[1])/dis, (max_point[2])/dis return step_x, step_y, step_z, ture_x, ture_y, ture_z 。这是我的函数的完整形式,报错的原因是TypeError: get_step() missing 2 required positional arguments: 'y' and 'z'
时间: 2024-02-18 21:00:05 浏览: 116
这个错误提示表明,在你调用 `get_step()` 函数时,你没有传入足够的参数。这很可能是因为你在调用函数时没有正确传递参数,导致函数期望接收到三个参数,但实际上只收到了一个或者没有参数。
请检查一下你调用 `get_step()` 函数的代码行,确保你传递了三个参数。例如:
```
x1, y1, z1 = map(int, input("Enter the values of x, y, z(space): ").split())
step_x, step_y, step_z, ture_x, ture_y, ture_z = get_step(x1, y1, z1)
```
在这个例子中,我们先使用 `map()` 函数从用户输入中获取三个整数,然后将这些整数作为参数传递给 `get_step()` 函数。最后,我们将 `get_step()` 函数的返回值存储到变量中。注意,我们需要使用六个变量来接收 `get_step()` 函数的返回值。
相关问题
def farthest_point_sample(xyz, npoint): """ Input: xyz: pointcloud data, [B, N, 3] npoint: number of samples Return: centroids: sampled pointcloud index, [B, npoint, 3] """ device = xyz.device B, N, C = xyz.shape centroids = torch.zeros(B, npoint, dtype=torch.long).to(device) # 采样点矩阵(B, npoint) distance = torch.ones(B, N).to(device) * 1e10 # 采样点到所有点距离(B, N) farthest = torch.randint(0, N, (B,), dtype=torch.long).to(device) # 最远点,初试时随机选择一点点 batch_indices = torch.arange(B, dtype=torch.long).to(device) # batch_size 数组 for i in range(npoint): centroids[:, i] = farthest # 更新第i个最远点 centroid = xyz[batch_indices, farthest, :].view(B, 1, 3) # 取出这个最远点的xyz坐标 dist = torch.sum((xyz - centroid) ** 2, -1) # 计算点集中的所有点到这个最远点的欧式距离 mask = dist < distance distance[mask] = dist[mask] # 更新distances,记录样本中每个点距离所有已出现的采样点的最小距离 farthest = torch.max(distance, -1)[1] # 返回最远点索引 return centroids 解释以上代码并说明需要的库
以上代码使用了PyTorch库来实现farthest point sampling(FPS)算法,用于从点云数据中均匀地采样一定数量的点。该算法首先随机选择一个点作为第一个采样点,然后计算所有点到这个采样点的距离,选择距离当前采样点最远的点作为下一个采样点,重复这个过程直到选出指定数量的采样点。
具体解释如下:
- 首先导入了PyTorch库,用于实现深度学习模型。
- farthest_point_sample函数中,xyz是输入的点云数据(B表示batch_size,N表示点云中点的数量,C表示每个点的坐标),npoint是需要采样的点的数量。
- 创建一个空的采样点矩阵centroids,用于存储选中的采样点的索引。
- 初始化距离矩阵distance,将其设置为一个非常大的值,用于存储每个点到所有已选采样点的最小距离。
- 随机选择一个点作为第一个采样点,将其赋值给变量farthest。
- 创建一个batch_size数组batch_indices,用于指定每个点所属的batch。
- 循环npoint次,每次选出距离当前采样点最远的点作为下一个采样点。
- 将当前采样点的索引farthest存储到采样点矩阵centroids中。
- 从点云数据xyz中取出当前采样点的坐标centroid。
- 计算所有点到当前采样点的欧式距离dist。
- 根据距离更新距离矩阵distance,记录样本中每个点距离所有已出现的采样点的最小距离。
- 选出距离所有已选采样点最远的点作为下一个采样点,更新变量farthest。
- 最终返回采样点矩阵centroids。
需要使用的库是PyTorch。
def point_cloud_template_matching(template, scene, threshold): """ 点云模板匹配算法 :param template: 模板点云,Nx3的numpy数组 :param scene: 场景点云,Mx3的numpy数组 :param threshold: 匹配阈值 :return: 匹配结果,Nx3的numpy数组,每行表示模板中的一个点在场景中的匹配点 """ # 构建KD树 tree = KDTree(scene) # 查询每个模板点的最近邻 dist, idx = tree.query(template) # 根据阈值筛选匹配点 matches = template[dist < threshold] return matches @numba.njit() def for_prodata(distances, num_samples, farthest_pts, points): for i in range(1, num_samples): # 选择距离已选点集合最远的点 farthest_pts[i] = points[np.argmax(distances)] # 更新距离 distances = np.minimum(distances, np.sqrt(((points - farthest_pts[i]) ** 2).sum(axis=1))) return farthest_pts解释这两段代码
这两段代码是点云模板匹配算法的实现,其中第一段代码实现了匹配过程,第二段代码实现了贪心算法中的迭代过程。
具体解释如下:
第一段代码中,定义了一个名为point_cloud_template_matching的函数,接收三个参数:模板点云template,场景点云scene和匹配阈值threshold。该函数首先使用场景点云构建了一个KD树,然后对于模板点云中的每个点,通过查询KD树找到场景点云中与其距离最近的点,并计算其距离。最后,根据设定的匹配阈值筛选出与模板点云中每个点匹配的场景点云中的点,并将这些点作为匹配结果返回。
第二段代码中,定义了一个名为for_prodata的函数,使用了@numba.njit()装饰器,表示使用Numba进行即时编译,优化代码的运行速度。该函数接收四个参数:距离distances、采样点数目num_samples、已选最远点集合farthest_pts和点云点集points。该函数的作用是在贪心算法中的每一轮迭代中,选择距离已选点集合最远的点,并更新距离。具体实现过程是,首先将第一个采样点作为已选点集合中的第一个点,然后对于每个后续采样点,选择距离已选点集合中的点最远的点作为新的采样点,并更新距离。最终,返回采样点集合farthest_pts。
阅读全文
相关推荐
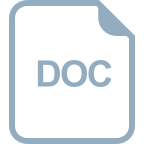
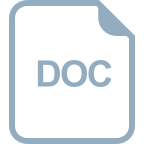
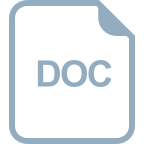
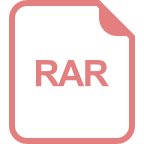
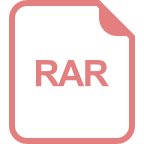
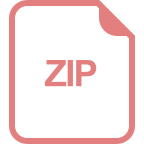
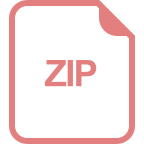









