优化修改代码,可以分系进行学历统计,计算各学历的人数:void countEducation() { Node* p = head->next; int bachelorCount = 0, masterCount = 0, doctoralCount = 0; while (p != nullptr) { if (p->data.getEducation() == "本科") { bachelorCount++; } else if (p->data.getEducation() == "硕士") { masterCount++; } else if (p->data.getEducation() == "博士") { doctoralCount++; } p = p->next; } cout << "本科:" << bachelorCount << endl; cout << "硕士:" << masterCount << endl; cout << "博士:" << doctoralCount << endl; }
时间: 2024-02-02 16:04:57 浏览: 30
可以使用一个 map 来记录各个学历对应的人数,然后遍历链表统计即可,这样可以避免大量的 if-else 判断,代码如下:
```c++
void countEducation() {
Node* p = head->next;
map<string, int> educationCount;
while (p != nullptr) {
educationCount[p->data.getEducation()]++;
p = p->next;
}
for (const auto& [education, count] : educationCount) {
cout << education << ":" << count << endl;
}
}
```
这样就可以统计出每个学历对应的人数,并且不需要对每个学历进行判断,代码更加简洁。
相关问题
调整以下代码删除节点:#include<stdio.h> #include<stdlib.h> typedef struct Node{ int data; Node* next; }Node; Node* createlist(){ Node* head=(Node*)malloc(sizeof(Node)); head->next=NULL; return head; } Node* createnode(int data){ Node* newnode=(Node*)malloc(sizeof(Node)); newnode->data=data; newnode->next=NULL; return newnode; } void insertnode(Node* head,int data){ Node* newnode=createnode(data); newnode->next=head->next; head->next=newnode; } void deletenode(Node* head){ Node* newnode=head->next; head->next=newnode->next->next; free(newnode); } void printlist(Node* head){ Node* p=head->next; while(p){ printf("%d",p->data); p=p->next; } printf("\n"); } int main() { Node* list=createlist(); insertnode(list,1); insertnode(list,3); insertnode(list,5); printlist(list); printf("\n"); deletenode(list); printlist(list); return 0; }
以下是已经删除节点的代码:
#include<stdio.h>
#include<stdlib.h>
typedef struct Node{
int data;
Node* next;
}Node;
Node* createlist(){
Node* head=(Node*)malloc(sizeof(Node));
head->next=NULL;
return head;
}
Node* createnode(int data){
Node* newnode=(Node*)malloc(sizeof(Node));
newnode->data=data;
newnode->next=NULL;
return newnode;
}
void insertnode(Node* head,int data){
Node* newnode=createnode(data);
newnode->next=head->next;
head->next=newnode;
}
void deletenode(Node* head){
Node* newnode=head->next;
head->next=newnode->next;
free(newnode);
}
void printlist(Node* head){
Node* p=head->next;
while(p){
printf("%d",p->data);
p=p->next;
}
printf("\n");
}
int main() {
Node* list=createlist();
insertnode(list,1);
insertnode(list,3);
insertnode(list,5);
printlist(list);
printf("\n");
deletenode(list);
printlist(list);
return 0;
}
优化修改代码,分别按照“系”进行学历统计,分别计算每个系中各学历的人数:void countEducation() { Node* p = head->next; int bachelorCount = 0, masterCount = 0, doctoralCount = 0; while (p != nullptr) { if (p->data.getEducation() == "本科") { bachelorCount++; } else if (p->data.getEducation() == "硕士") { masterCount++; } else if (p->data.getEducation() == "博士") { doctoralCount++; } p = p->next; } cout << "本科:" << bachelorCount << endl; cout << "硕士:" << masterCount << endl; cout << "博士:" << doctoralCount << endl; }
可以使用一个嵌套的 map 来记录各系对应的各个学历的人数,然后遍历链表统计即可,代码如下:
```c++
void countEducation() {
Node* p = head->next;
map<string, map<string, int>> educationCount; // 用 map 记录各个系对应的各个学历的人数
while (p != nullptr) {
educationCount[p->data.getDepartment()][p->data.getEducation()]++;
p = p->next;
}
for (const auto& [department, educationMap] : educationCount) {
cout << department << "系:" << endl;
for (const auto& [education, count] : educationMap) {
cout << education << ":" << count << endl;
}
cout << endl;
}
}
```
这样就可以统计出每个系中各个学历的人数,输出格式也更加清晰。
相关推荐
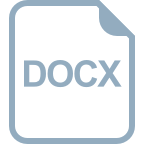
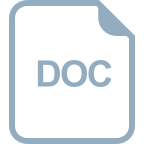
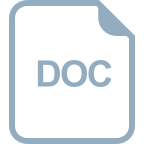













