void Inverse(struct node* head) { struct node* p = head; struct node* q = head; struct node* m = head; while (p->next) { p = p->next; if (q == head) q->next = NULL; m = p->next; p->next = q; m->next = p; q = m; p = m; } p->next = q; }
时间: 2024-04-12 22:26:35 浏览: 85
您提供的代码存在一些问题,无法正确地完成链表的逆置。在代码中,您使用了三个指针变量p、q和m来进行链表节点的重新连接。但是,您在更新节点的指针时出现了一些错误。
以下是对您提供的代码进行修正后的版本:
```c
void Inverse(struct node* head) {
struct node* prev = NULL;
struct node* curr = head;
struct node* next = NULL;
while (curr != NULL) {
next = curr->next; // 保存下一个节点
curr->next = prev; // 当前节点指向前一个节点
prev = curr; // 前一个节点更新为当前节点
curr = next; // 当前节点更新为下一个节点
}
// 更新链表的头节点
head = prev;
}
```
修正后的代码使用了三个指针变量prev、curr和next来进行节点的重新连接。循环遍历链表,每次将当前节点的指针指向前一个节点,并依次更新三个指针变量。最后,将链表的头节点更新为prev,以完成链表的逆置操作。
请注意,在修正后的代码中,我将函数参数名从p、q和m更改为了prev、curr和next,以提高代码的可读性。另外,请确保在调用该函数时传入正确的链表头节点。
相关问题
#include<stdio.h> #include<math.h> #include<stdlib.h> #define dx 100 struct bb { int m; int n; int hl[dx][dx]; int jk[dx][dx]; }; double det(struct bb *A, int n); double algebraic_cofactor(struct bb *A, struct bb *B, int row, int col); void adjoint(struct bb *A, struct bb *B); void inverse(struct bb *A,double inv[][dx],int N); int main() { struct bb A; int m,n; printf("输入几行几列:\n"); scanf("%d %d",&m,&n); A.m = m; A.n = n; printf("请输入矩阵:\n"); for(int i = 0; i < A.m; i++) { for(int j = 0; j < A.n; j++) { scanf("%d", &A.hl[i][j]); } } double inv[dx][dx]; int N = A.m; // Assuming square matrix // 计算逆矩阵 inverse(&A, inv, N); // 输出结果 printf("逆矩阵:\n"); for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { printf("%.2f ", A.hl[i][j]); } printf("\n"); } return 0; } double det(struct bb *A, int n) { double sum=0; if(n==1) { return A->hl[0][0]; } else if(n==2) { return A->hl[0][0]*A->hl[1][1]-A->hl[0][1]*A->hl[1][0]; } int i,j,k; struct bb *mybb = (struct bb *)malloc(sizeof(struct bb)); for(k=0;k<n;k++) { double b[dx][dx]; for(i=1;i<n;i++) { for(j=0;j<n;j++) { if(j<k) { b[i-1][j]=A->hl[i][j]; } else if(j>k) { b[i-1][j-1]=A->hl[i][j]; } } } mybb->m = n - 1; mybb->n = n - 1; for(i=0;i<mybb->m;i++) { for(j=0;j<mybb->n;j++) { mybb->hl[i][j] = b[i][j]; } } double detb=det(mybb,n-1); sum+=A->hl[0][k]*pow(-1,k)*detb; } free(mybb); return sum; } double algebraic_cofactor(struct bb *A, struct bb B, int row, int col) { int i,j,m=0,n=0,M=A->m; double sign; if((row+col)%2==0) { sign=1; } else { sign=-1; } for(i=0;i<M;i++) { if(i!=row) { for(j=0;j<M;j++) { if(j!=col) { B->jk[m][n]=A->hl[i][j]; n++; } } m++; n=0; } } double detb=det(B,M-1); return signdetb; } void adjoint(struct bb *A, struct bb *B) { int i,j,M=A->m; for(i=0;i<M;i++) { for(j=0;j<M;j++) { B->hl[j][i]=algebraic_cofactor(A,B,i,j); //注意这里是 hl[j][i] 而不是 hl[i][j] } } } void inverse(struct bb *A,double inv[][dx],int N) { // 构造伴随矩阵 struct bb B; B.m = N; B.n = N; adjoint(A, &B); // 计算行列式的值 double dets=det(A,N); // 判断行列式是否为零 if(dets == 0) { printf("该矩阵不可逆!\n"); return; } // 计算逆矩阵 for(int i=0;i<N;i++) { for(int j=0;j<N;j++) { inv[i][j] = B.hl[i][j] / dets; } } }修改这个代码找出错误并改正
在函数`algebraic_cofactor`中,参数B应该是一个指向结构体`bb`的指针,因为在函数内部需要对B进行修改,而如果是结构体B,则只是传值,无法修改原来的B。所以需要将函数定义改为:
```
double algebraic_cofactor(struct bb *A, struct bb *B, int row, int col)
```
然后在函数内部将`B->jk[m][n]`改为`(*B).jk[m][n]`或者`B->jk[m][n]`改为`B[0].jk[m][n]`,这是因为B是一个指向结构体的指针,需要通过指针访问结构体的成员变量。
写出该调用函数的后续代码void extendedEuclid(e, (p - 1) * (q - 1), &d)
这似乎是在描述使用扩展欧几里得算法(Extended Euclidean Algorithm)计算两个大素数 p 和 q 的最大公约数(GCD),其中 e 是模指数,`(p - 1) * (q - 1)` 是模 n 的逆元乘积,而 `&d` 指向一个整数变量 d,用于存储结果。
在 C 语言中,如果你已经有了 `extendedEuclid` 函数原型,其大致结构可能是这样的:
```c
// 假设 extendedEuclid 函数声明已存在
typedef struct {
int a; // 最终 gcd
int x;
int y;
} ExtendedEuclidResult;
ExtendedEuclidResult extendedEuclid(int e, int n, int* d); // 返回 GCD、x 及 y
int main() {
int p = ...; // 大素数 p
int q = ...; // 另一大素数 q
int d = 0; // 存储 d 的地方,初始化为0
// 计算模 n 的逆元乘积,这里假设 n = (p - 1) * (q - 1)
int product_inverse = (p - 1) * (q - 1);
// 调用 extendedEuclid 函数,并将 d 的地址传入
ExtendedEuclidResult result = extendedEuclid(e, product_inverse, &d);
// 获取 GCD 和 x, y 的值
int gcd = result.a;
int x = result.x;
int y = result.y;
// 如果需要,可以打印结果或进一步使用它们
printf("GCD of %d and %d is %d\n", e, product_inverse, gcd);
printf("x = %d, y = %d\n", x, y);
return 0;
}
```
阅读全文
相关推荐
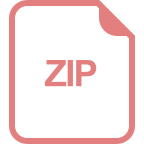
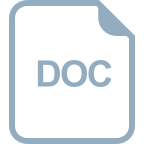
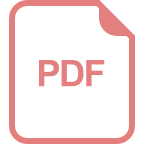













