购物车页面可实现商品数量的增减、商品的删除以及总价格计算功能,和item界面。用Android实现写出代码
时间: 2024-03-04 15:50:30 浏览: 163
好的,这是一个比较复杂的问题,我会尽可能地为您提供一些思路和代码示例。首先,我们需要创建一个购物车页面的布局文件,可以使用RecyclerView来展示购物车中的商品列表,同时需要添加一些控件来显示总价格和结算按钮。以下是一个简单的购物车布局文件示例:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<!-- 商品列表 -->
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" />
<!-- 总价格和结算按钮 -->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="16dp">
<TextView
android:id="@+id/total_price_textview"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="总价:0元" />
<Button
android:id="@+id/checkout_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="结算" />
</LinearLayout>
</LinearLayout>
```
接下来,我们需要创建一个Adapter来将商品列表展示在RecyclerView中。这个Adapter可以继承自RecyclerView.Adapter,并且需要实现一些必要的方法,例如创建ViewHolder和绑定数据到ViewHolder中。以下是一个简单的购物车Adapter示例:
```java
public class ShoppingCartAdapter extends RecyclerView.Adapter<ShoppingCartAdapter.ViewHolder> {
private List<Product> mProducts;
private OnItemClickListener mListener;
public ShoppingCartAdapter(List<Product> products, OnItemClickListener listener) {
mProducts = products;
mListener = listener;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.item_shopping_cart, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
Product product = mProducts.get(position);
holder.nameTextView.setText(product.getName());
holder.priceTextView.setText(String.format("%d元", product.getPrice()));
holder.quantityTextView.setText(String.valueOf(product.getQuantity()));
holder.addButton.setOnClickListener(v -> mListener.onAddButtonClick(position));
holder.minusButton.setOnClickListener(v -> mListener.onMinusButtonClick(position));
holder.deleteButton.setOnClickListener(v -> mListener.onDeleteButtonClick(position));
}
@Override
public int getItemCount() {
return mProducts.size();
}
public static class ViewHolder extends RecyclerView.ViewHolder {
public TextView nameTextView;
public TextView priceTextView;
public TextView quantityTextView;
public Button addButton;
public Button minusButton;
public Button deleteButton;
public ViewHolder(View itemView) {
super(itemView);
nameTextView = itemView.findViewById(R.id.name_textview);
priceTextView = itemView.findViewById(R.id.price_textview);
quantityTextView = itemView.findViewById(R.id.quantity_textview);
addButton = itemView.findViewById(R.id.add_button);
minusButton = itemView.findViewById(R.id.minus_button);
deleteButton = itemView.findViewById(R.id.delete_button);
}
}
public interface OnItemClickListener {
void onAddButtonClick(int position);
void onMinusButtonClick(int position);
void onDeleteButtonClick(int position);
}
}
```
在上面的代码中,我们通过实现ViewHolder来缓存商品的视图,然后在onBindViewHolder方法中将商品的信息绑定到ViewHolder中。我们还添加了一些按钮来实现商品数量的增减和商品的删除操作,并通过接口来回调这些操作。
接下来,我们需要在Activity中创建一个购物车实例,并将其绑定到RecyclerView中。同时,我们还需要实现Adapter中定义的OnItemClickListener接口,来处理商品数量的增减和商品的删除操作。以下是一个简单的购物车Activity示例:
```java
public class ShoppingCartActivity extends AppCompatActivity implements ShoppingCartAdapter.OnItemClickListener {
private List<Product> mProducts;
private RecyclerView mRecyclerView;
private ShoppingCartAdapter mAdapter;
private TextView mTotalPriceTextView;
private Button mCheckoutButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_shopping_cart);
// 初始化商品列表
mProducts = new ArrayList<>();
mProducts.add(new Product("商品1", 10, 1));
mProducts.add(new Product("商品2", 20, 2));
mProducts.add(new Product("商品3", 30, 3));
// 初始化RecyclerView
mRecyclerView = findViewById(R.id.recycler_view);
mAdapter = new ShoppingCartAdapter(mProducts, this);
mRecyclerView.setAdapter(mAdapter);
mRecyclerView.setLayoutManager(new LinearLayoutManager(this));
// 初始化总价格和结算按钮
mTotalPriceTextView = findViewById(R.id.total_price_textview);
mCheckoutButton = findViewById(R.id.checkout_button);
mCheckoutButton.setOnClickListener(v -> {
// TODO: 处理结算操作
});
// 更新总价格
updateTotalPrice();
}
@Override
public void onAddButtonClick(int position) {
// 增加商品数量
mProducts.get(position).increaseQuantity();
mAdapter.notifyItemChanged(position);
// 更新总价格
updateTotalPrice();
}
@Override
public void onMinusButtonClick(int position) {
// 减少商品数量
mProducts.get(position).decreaseQuantity();
mAdapter.notifyItemChanged(position);
// 更新总价格
updateTotalPrice();
}
@Override
public void onDeleteButtonClick(int position) {
// 删除商品
mProducts.remove(position);
mAdapter.notifyItemRemoved(position);
// 更新总价格
updateTotalPrice();
}
private void updateTotalPrice() {
// 计算总价格
int totalPrice = 0;
for (Product product : mProducts) {
totalPrice += product.getPrice() * product.getQuantity();
}
// 更新总价格视图
mTotalPriceTextView.setText(String.format("总价:%d元", totalPrice));
}
}
```
在上面的代码中,我们首先初始化商品列表,并将其传递给购物车Adapter。接着,我们实现了Adapter中定义的OnItemClickListener接口,来处理商品数量的增减和商品的删除操作。我们还添加了一个updateTotalPrice方法来计算总价格,并更新总价格视图。
以上是一个简单的购物车页面的实现示例,您可以根据您的需求进行修改和扩展。
阅读全文
相关推荐
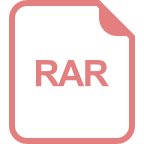







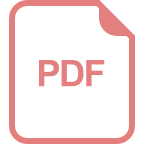
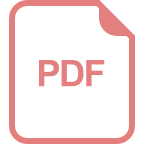
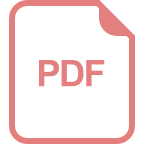
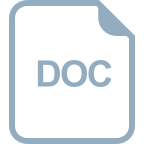




