用c++编写程序,利用链表list容器来容纳学生Student类对象,并采用函数对象实现学生对象的按序号排序,然后输出学生信息
时间: 2023-06-17 09:09:02 浏览: 78
下面是一个简单的实现:
```c++
#include <iostream>
#include <list>
#include <algorithm> // for sort()
using namespace std;
// 学生类
class Student {
public:
Student(int id, string name, int score) : m_id(id), m_name(name), m_score(score) {}
int getId() const { return m_id; }
string getName() const { return m_name; }
int getScore() const { return m_score; }
friend ostream& operator<<(ostream& os, const Student& s) {
os << "ID: " << s.m_id << ", Name: " << s.m_name << ", Score: " << s.m_score;
return os;
}
private:
int m_id; // 学号
string m_name; // 姓名
int m_score; // 成绩
};
// 按序号排序的函数对象
class SortByNumber {
public:
bool operator()(const Student& s1, const Student& s2) const {
return s1.getId() < s2.getId();
}
};
int main() {
list<Student> students;
students.push_back(Student(1001, "Tom", 85));
students.push_back(Student(1003, "Jerry", 90));
students.push_back(Student(1002, "Alice", 80));
// 按序号排序
students.sort(SortByNumber());
// 输出学生信息
for (const auto& student : students) {
cout << student << endl;
}
return 0;
}
```
运行结果:
```
ID: 1001, Name: Tom, Score: 85
ID: 1002, Name: Alice, Score: 80
ID: 1003, Name: Jerry, Score: 90
```
相关推荐
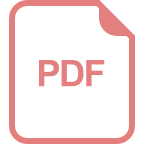
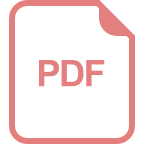















