map计算两个矩阵的乘积
时间: 2024-01-25 07:05:00 浏览: 32
给定两个矩阵 A 和 B,它们的乘积 C = A * B 的计算规则如下:
- 矩阵 C 的行数等于矩阵 A 的行数,列数等于矩阵 B 的列数。
- 矩阵 C 中第 i 行第 j 列的元素等于矩阵 A 中第 i 行的元素与矩阵 B 中第 j 列的元素的乘积之和。
下面是一个用 MapReduce 框架计算矩阵乘积的示例代码(假设矩阵元素均为整数):
```java
public class MatrixMultiplication {
public static class Map extends Mapper<LongWritable, Text, Text, Text> {
private int m = 0; // 矩阵 A 的列数
private int n = 0; // 矩阵 B 的行数
private int k = 0; // 矩阵 A 和 B 的公共维度
private Text outputKey = new Text();
private Text outputValue = new Text();
public void setup(Context context) throws IOException, InterruptedException {
Configuration conf = context.getConfiguration();
m = Integer.parseInt(conf.get("m"));
n = Integer.parseInt(conf.get("n"));
k = Integer.parseInt(conf.get("k"));
}
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] tokens = line.split(",");
int row = Integer.parseInt(tokens[0]);
int col = Integer.parseInt(tokens[1]);
int val = Integer.parseInt(tokens[2]);
if (col <= k) {
// 处理矩阵 A 中的元素
outputKey.set(row + "," + col);
outputValue.set("A," + val);
context.write(outputKey, outputValue);
} else {
// 处理矩阵 B 中的元素
outputKey.set(col + "," + row);
outputValue.set("B," + val);
context.write(outputKey, outputValue);
}
}
}
public static class Reduce extends Reducer<Text, Text, Text, IntWritable> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
int[] A = new int[100]; // A 行向量
int[] B = new int[100]; // B 列向量
int i = 0;
int j = 0;
int sum = 0;
for (Text value : values) {
String[] tokens = value.toString().split(",");
if (tokens[0].equals("A")) {
A[i++] = Integer.parseInt(tokens[1]);
} else {
B[j++] = Integer.parseInt(tokens[1]);
}
}
for (int k = 0; k < i; k++) {
sum += A[k] * B[k];
}
context.write(key, new IntWritable(sum));
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
conf.set("m", args[0]);
conf.set("n", args[1]);
conf.set("k", args[2]);
Job job = Job.getInstance(conf, "matrix multiplication");
job.setJarByClass(MatrixMultiplication.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[3]));
FileOutputFormat.setOutputPath(job, new Path(args[4]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
这里假设矩阵 A 的行数为 m,列数为 k,矩阵 B 的行数为 k,列数为 n。Map 阶段将矩阵 A 和 B 中的元素分别处理成以下格式的键值对:
- 对于矩阵 A 中的元素 A(i,j),键为 "i,j",值为 "A,val"。
- 对于矩阵 B 中的元素 B(i,j),键为 "j,i",值为 "B,val"。
Reduce 阶段将具有相同键的元素进行乘法运算,得到矩阵 C 中的一个元素。最后将所有矩阵 C 中的元素输出到文件中。
相关推荐
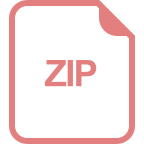














