设计一个按时间片轮转法实现处理器调度的程序
时间: 2023-04-27 12:00:25 浏览: 113
好的,以下是一个使用时间片轮转法实现处理器调度的程序示例(使用Python语言编写):
```python
# 定义进程类
class Process:
def __init__(self, name, burst_time):
self.name = name # 进程名
self.burst_time = burst_time # 进程需要的CPU时间
self.remaining_time = burst_time # 剩余需要的CPU时间
# 执行进程
def execute(self, time_slice):
if self.remaining_time > time_slice:
self.remaining_time -= time_slice
print(f"{self.name} 执行了 {time_slice} 个时间片,剩余需要的CPU时间为 {self.remaining_time}")
else:
print(f"{self.name} 执行完毕!")
self.remaining_time = 0
# 时间片轮转法调度函数
def round_robin(processes, time_slice):
ready_queue = processes.copy() # 将所有进程添加到就绪队列中
while len(ready_queue) > 0:
# 从就绪队列中取出第一个进程
current_process = ready_queue.pop(0)
# 执行当前进程
current_process.execute(time_slice)
# 如果当前进程还需要执行,将其添加到就绪队列尾部
if current_process.remaining_time > 0:
ready_queue.append(current_process)
# 如果当前进程已经执行完毕,从进程列表中删除
else:
processes.remove(current_process)
# 测试程序
if __name__ == '__main__':
# 创建进程列表
processes = [Process("P1", 10), Process("P2", 5), Process("P3", 7)]
# 使用时间片轮转法调度进程
round_robin(processes, 2)
```
该程序首先定义了一个进程类 `Process`,包含进程名、需要的CPU时间以及剩余需要的CPU时间等属性,以及一个 `execute` 方法用于执行进程。然后定义了一个 `round_robin` 函数,该函数接收一个进程列表和一个时间片大小作为参数,使用时间片轮转法调度进程。最后在 `__name__ == '__main__'` 的条件下,创建一个进程列表并调用 `round_robin` 函数进行测试。
在测试时,程序会按照进程列表中的进程顺序依次执行,每个进程执行的时间为一个时间片(这里设置为2个时间片),直到所有进程执行完毕。程序会输出每个进程执行的时间以及剩余需要的CPU时间。
相关推荐
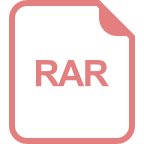
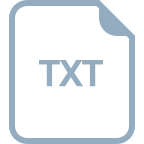
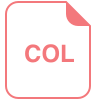












