if(usart2_data==2&&usart2_data!=99)//发送2时 { printf("值为%d\r\n",usart2_data); LED0=0; LED1=0; usart2_data=99; } else if(usart2_data==0&&usart2_data!=99)//发送0时 { printf("值为%d\r\n",usart2_data); LED0=1; LED1=1; usart2_data=99;
时间: 2024-04-04 12:33:54 浏览: 51
这段代码看起来是针对串口通信的,当接收到数据为2时,会将 LED0 和 LED1 置为低电平,同时将 usart2_data 设为99,表示已经处理完数据;当接收到数据为0时,会将 LED0 和 LED1 置为高电平,同时将 usart2_data 设为99,表示已经处理完数据。不过需要注意的是,这段代码没有对 usart2_data 进行赋值,需要在其他地方对其进行初始化或者赋值操作。
相关问题
while(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET); RX_Data = USART_ReceiveData(USART1); if(RX_Data == '1') { Go(); printf("Go"); } else if(RX_Data == '2') { Back(); printf("Back"); } else if(RX_Data == '3') { Left(); printf("Left"); } else if(RX_Data == '4') { Right(); printf("Right"); } else { Stop(); printf("Stop");
看起来这是一段基于USART通信的代码,它通过检查USART1接收寄存器中是否有数据来判断是否接收到数据,并根据不同的接收数据执行相应的操作。当接收到字符'1'时,执行Go()函数,并通过printf()函数输出"Go";当接收到字符'2'时,执行Back()函数,并输出"Back";当接收到字符'3'时,执行Left()函数,并输出"Left";当接收到字符'4'时,执行Right()函数,并输出"Right";否则执行Stop()函数,并输出"Stop"。
#include "stm32f10x.h"#include "stdio.h"#define RX_BUFFER_SIZE 9uint8_t rx_buffer[RX_BUFFER_SIZE];uint8_t rx_index = 0;void USART1_Init(void){ GPIO_InitTypeDef GPIO_InitStructure; USART_InitTypeDef USART_InitStructure; // 打开USART1和GPIOA时钟 RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE); // 配置USART1的GPIO引脚 GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(GPIOA, &GPIO_InitStructure); GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; GPIO_Init(GPIOA, &GPIO_InitStructure); // 配置USART1的通信参数 USART_InitStructure.USART_BaudRate = 9600; USART_InitStructure.USART_WordLength = USART_WordLength_8b; USART_InitStructure.USART_StopBits = USART_StopBits_1; USART_InitStructure.USART_Parity = USART_Parity_No; USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; USART_Init(USART1, &USART_InitStructure); // 打开USART1 USART_Cmd(USART1, ENABLE);}void USART1_IRQHandler(void){ if (USART_GetITStatus(USART1, USART_IT_RXNE) == SET) { uint8_t data = USART_ReceiveData(USART1); if (rx_index < RX_BUFFER_SIZE) { rx_buffer[rx_index++] = data; } if (rx_index == RX_BUFFER_SIZE) { USART_ITConfig(USART1, USART_IT_RXNE, DISABLE); } }}int main(void){ USART1_Init(); while (1) { // 发送查询指令 USART_SendData(USART1, 0xFF); USART_SendData(USART1, 0x01); USART_SendData(USART1, 0x86); USART_SendData(USART1, 0x00); USART_SendData(USART1, 0x00); USART_SendData(USART1, 0x00); USART_SendData(USART1, 0x00); USART_SendData(USART1, 0x00); USART_SendData(USART1, 0x79); // 等待数据接收完成 rx_index = 0; USART_ITConfig(USART1, USART_IT_RXNE, ENABLE); while (rx_index < RX_BUFFER_SIZE); // 计算甲醛浓度 uint16_t ch2o_raw = (rx_buffer[2] << 8) | rx_buffer[3]; float ch2o_conc = ch2o_raw / 1000.0; // 显示甲醛浓度 printf("CH2O Concentration: %.3f mg/m3\r\n", ch2o_conc); // 等待一段时间后再进行下一次检测 delay_ms(1000); }}void delay_ms(uint32_t ms){ uint32_t i, j; for (i = 0; i < ms; i++) for (j = 0; j < 2000; j++);}代码中串口是不是错了,应该是usart3吧
是的,你说得对,代码中的串口定义应该改为USART3,如下所示:
```c
#include "stm32f10x.h"
#include "stdio.h"
#define RX_BUFFER_SIZE 9
uint8_t rx_buffer[RX_BUFFER_SIZE];
uint8_t rx_index = 0;
void USART3_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 打开USART3和GPIOB时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART3, ENABLE);
// 配置USART3的GPIO引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_11;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// 配置USART3的通信参数
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure
阅读全文
相关推荐
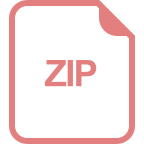
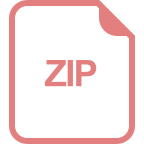
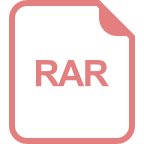














