c++ dlib 用 resnet-34 实现人脸检测
时间: 2023-11-28 17:47:28 浏览: 62
使用 C++ 和 dlib 库实现人脸检测是非常简单的。dlib 库已经内置了 resnet-34 模型,可以直接用于人脸检测。
以下是一个简单的示例代码:
```c++
#include <iostream>
#include <dlib/opencv.h>
#include <opencv2/opencv.hpp>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
using namespace std;
using namespace dlib;
using namespace cv;
int main()
{
try
{
// 初始化人脸检测器
frontal_face_detector detector = get_frontal_face_detector();
// 初始化形状预测器,用于获取人脸关键点
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
// 加载图像
Mat img = imread("test.jpg");
// 将图像转换为 dlib 的图像格式
cv_image<bgr_pixel> cimg(img);
// 进行人脸检测
std::vector<dlib::rectangle> faces = detector(cimg);
// 遍历检测到的人脸
for (size_t i = 0; i < faces.size(); ++i)
{
// 获取人脸关键点
full_object_detection shape = sp(cimg, faces[i]);
// 在图像上绘制人脸矩形框和关键点
for (unsigned long j = 0; j < shape.num_parts(); ++j)
{
circle(img, cv::Point(shape.part(j).x(), shape.part(j).y()), 2, cv::Scalar(0, 255, 0), -1);
}
rectangle(img, cv::Point(faces[i].left(), faces[i].top()), cv::Point(faces[i].right(), faces[i].bottom()), cv::Scalar(0, 0, 255), 2);
}
// 显示结果
imshow("result", img);
waitKey(0);
}
catch (exception& e)
{
cout << e.what() << endl;
}
return 0;
}
```
这个示例代码中,我们首先加载了一张名为 "test.jpg" 的图像。然后使用 dlib 的人脸检测器进行人脸检测,并获取人脸关键点。最后,在图像上绘制人脸矩形框和关键点,并显示结果。
需要注意的是,我们使用的是 dlib 提供的形状预测器,该预测器可以预测出人脸的 68 个关键点。如果你想要使用自己的形状预测器或者检测更多的关键点,需要对代码进行相应的修改。
相关推荐
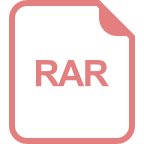
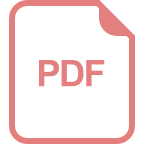
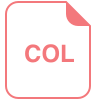
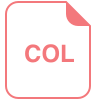

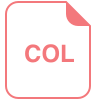
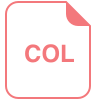









