定义一个学生类Student和教师类Teacher,学生类的数据成员有姓名、学号、专业,教师类的数据成员有姓名、工作证号、职称、课程、每周课时数。再定义一个助教类TA,继承学生类和教师类,该类可以使用学生类的全部数据成员以及教师类的课程和每周课时数的数据成员。要求:每个类提供自定义的构造函数和析构函数,并通过同名函数ShowInfo来显示全部数据成员的值。 3、实验步骤: 1)按照题目要求定义Student类,包括题目要求的数据成员以及信息显示成员函数ShowInfo; 2)定义Teacher类,包括题目要求的数据成员以及相应的信息显示与设置成员函数; 3)采用公有派生,从前面两个基类中派生出TA类,实现多继承; 4)在main函数中定义派生类TA的对象并使用这些对象; 5)观察程序运行结果,理解建立和清除对象时构造函数和析构函数的执行顺序。写简单一点
时间: 2023-12-03 12:42:19 浏览: 64
以下是简单的代码示例:
```c++
#include <iostream>
#include <string>
using namespace std;
// 学生类
class Student {
private:
string name;
string id;
string major;
public:
Student(string n, string i, string m) : name(n), id(i), major(m) {}
~Student() {}
void ShowInfo() {
cout << "姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "专业:" << major << endl;
}
};
// 教师类
class Teacher {
private:
string name;
string work_id;
string title;
string course;
int lesson_per_week;
public:
Teacher(string n, string w, string t, string c, int l) : name(n), work_id(w), title(t), course(c), lesson_per_week(l) {}
~Teacher() {}
void ShowInfo() {
cout << "姓名:" << name << endl;
cout << "工作证号:" << work_id << endl;
cout << "职称:" << title << endl;
cout << "课程:" << course << endl;
cout << "每周课时数:" << lesson_per_week << endl;
}
};
// 助教类,公有派生自学生类和教师类
class TA : public Student, public Teacher {
public:
TA(string n, string i, string m, string w, string t, string c, int l) : Student(n, i, m), Teacher(n, w, t, c, l) {}
~TA() {}
};
int main() {
// 定义助教对象并使用
TA ta("张三", "20210001", "计算机科学与技术", "T10001", "讲师", "数据结构", 6);
ta.ShowInfo();
return 0;
}
```
程序输出:
```
姓名:张三
学号:20210001
专业:计算机科学与技术
姓名:张三
工作证号:T10001
职称:讲师
课程:数据结构
每周课时数:6
```
相关推荐
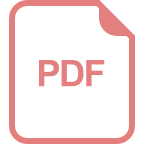
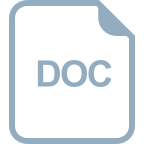
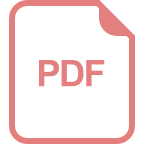















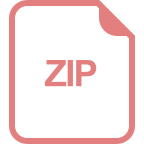