运用MATLAB对支持向量机进行编程,依据样本数据进行失效二分类判断
时间: 2023-03-08 10:36:39 浏览: 57
很高兴为您解答,MATLAB 编程对支持向量机的失效二分类判断是比较复杂的,需要您掌握相关的数学基础,包括有线性代数、概率论和统计等知识,以便理解支持向量机模型的原理。同时,您还需要熟练掌握 MATLAB 语言,以便对支持向量机模型进行编程。
相关问题
用MCMC生成样本并且用子集模拟计算失效概率matlab实现
下面是一个用MCMC生成样本并用子集模拟计算失效概率的MATLAB实现:
首先,我们需要定义一个函数来计算失效概率。假设我们有一个包含n个元素的系统,并且每个元素的失效概率为p。我们可以使用二项分布来计算失效概率:
```matlab
function p_failure = calculate_failure_probability(n, p, subset)
% n: total number of elements in the system
% p: failure probability of each element
% subset: a binary vector indicating which elements are included in the subset
% calculate the probability that at least one element fails in the subset
p_subset = 1 - prod((1 - p) .^ subset);
% calculate the probability that no other element fails in the system
p_other = prod((1 - subset) + subset .* (1 - p));
% calculate the overall failure probability
p_failure = p_subset * p_other;
end
```
现在我们可以使用MCMC生成样本。我们从一个随机的二元向量开始,并进行迭代。在每次迭代中,我们随机选择一个元素并翻转它的值。如果新的向量的失效概率更低,则接受该向量;否则,以一定的概率接受它,以便我们可以跳出局部极小值。
```matlab
function samples = generate_samples(n, p, num_samples, burn_in, thinning)
% n: total number of elements in the system
% p: failure probability of each element
% num_samples: number of samples to generate
% burn_in: number of iterations for burn-in period
% thinning: thinning factor for samples
% initialize a random binary vector as the starting point
current = rand(n, 1) < p;
% generate samples using MCMC
samples = false(n, num_samples);
for i = 1:(num_samples * thinning + burn_in)
% randomly choose an element to flip
index = randi(n);
proposal = current;
proposal(index) = ~proposal(index);
% calculate the acceptance probability
p_current = calculate_failure_probability(n, p, current);
p_proposal = calculate_failure_probability(n, p, proposal);
acceptance_prob = min(1, p_proposal / p_current);
% accept or reject the proposal
if rand < acceptance_prob
current = proposal;
end
% record the current state as a sample
if i > burn_in && mod(i - burn_in, thinning) == 0
samples(:, (i - burn_in) / thinning) = current;
end
end
end
```
最后,我们可以使用这些样本来估计失效概率。我们可以选择一些样本,并使用它们的平均值来估计失效概率。我们还可以计算置信区间,以了解我们的估计的准确性。
```matlab
function [p_failure, conf_interval] = estimate_failure_probability(n, p, samples, confidence)
% n: total number of elements in the system
% p: failure probability of each element
% samples: binary matrix containing the generated samples
% confidence: confidence level for the confidence interval
% calculate the failure probability for each sample
p_samples = zeros(size(samples, 2), 1);
for i = 1:size(samples, 2)
p_samples(i) = calculate_failure_probability(n, p, samples(:, i));
end
% estimate the failure probability and confidence interval
p_failure = mean(p_samples);
conf_interval = quantile(p_samples, [(1 - confidence) / 2, (1 + confidence) / 2]);
end
```
现在我们可以使用这些函数来生成样本并估计失效概率。例如,我们可以使用以下代码来生成10000个样本,然后使用其中的1000个样本来估计失效概率:
```matlab
n = 10; % number of elements in the system
p = 0.1; % failure probability of each element
num_samples = 10000;
burn_in = 1000;
thinning = 10;
samples = generate_samples(n, p, num_samples, burn_in, thinning);
subset_samples = samples(:, 1:1000);
[p_failure, conf_interval] = estimate_failure_probability(n, p, subset_samples, 0.95);
disp(['Failure probability: ', num2str(p_failure)]);
disp(['Confidence interval: [', num2str(conf_interval(1)), ', ', num2str(conf_interval(2)), ']']);
```
目标函数y=x(1)*x(2)-1500;mu=[38 54];sigma=[3.8 2.7] ,用MCMC生成样本并且用子集模拟计算失效概率matlab实现
以下是实现步骤:
1. 首先,我们需要用MCMC生成样本。我们可以使用Metropolis-Hastings算法来进行MCMC采样。具体步骤如下:
- 首先,我们需要定义一个初始状态,即一个二元向量x=[x(1), x(2)],并且需要设定一个步长h,用于随机地生成新状态。
- 接着,我们需要计算当前状态x的目标函数值y,即y=x(1)*x(2)-1500。
- 然后,我们随机生成一个新状态x_new=[x_new(1), x_new(2)],其中x_new(1)~N(x(1), h)且x_new(2)~N(x(2), h)。这里,N表示正态分布。
- 计算新状态的目标函数值y_new。
- 计算接受概率p=min(1, exp(-(y_new-y)),其中exp为指数函数。如果p>=rand,即一个0到1之间的随机数,我们就接受新状态,否则就保留旧状态。
- 重复上述步骤,直到达到所需的样本量。
下面是Matlab代码实现:
```
mu = [38, 54];
sigma = [3.8, 2.7];
h = 1;
N = 10000; % 样本量
x = zeros(N, 2);
y = zeros(N, 1);
x(1,:) = mu;
y(1) = x(1,1)*x(1,2)-1500;
for i = 2:N
x_new = x(i-1,:) + h*randn(1,2);
y_new = x_new(1)*x_new(2)-1500;
p = min(1, exp(-(y_new-y(i-1))));
if p >= rand
x(i,:) = x_new;
y(i) = y_new;
else
x(i,:) = x(i-1,:);
y(i) = y(i-1);
end
end
```
2. 接下来,我们需要用子集模拟来估计失效概率。具体步骤如下:
- 首先,我们需要将目标函数转化为失效函数,即g(x)=max(0, 1500-x(1)*x(2))。这里,g(x)表示当目标函数小于等于1500时的失效概率。
- 然后,我们随机生成一些样本,这些样本应该符合初始状态的分布。
- 对于每个样本,我们计算其失效函数值,并将其与一个随机数比较。如果失效函数值大于随机数,则认为该样本失效。
- 重复上述步骤,直到达到所需的样本量。
- 最后,失效概率可以估计为失效样本数除以总样本数。
下面是Matlab代码实现:
```
g = @(x) max(0, 1500-x(1)*x(2));
M = 100000; % 总样本量
N_sub = 1000; % 子集大小
x_init = mu;
x_sub = mvnrnd(x_init, diag(sigma.^2), N_sub);
g_sub = zeros(N_sub, 1);
fail_count = 0;
for i = 1:N_sub
g_sub(i) = g(x_sub(i,:));
if g_sub(i) >= rand
fail_count = fail_count + 1;
end
end
p_fail = fail_count / N_sub;
```
注意,这里我们只使用了一个子集。在实际应用中,为了减小估计误差,可能需要使用多个子集。
相关推荐
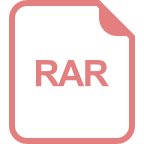
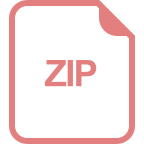
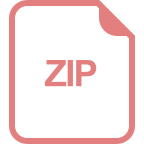






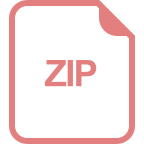
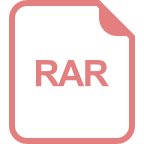
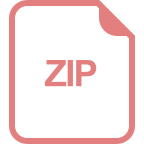
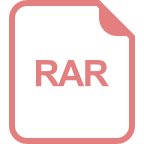
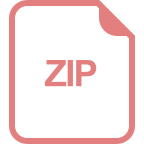
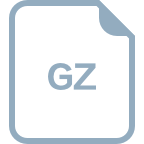
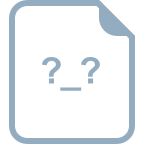