LSTNet模型的代码
时间: 2024-03-20 17:20:37 浏览: 18
下面是使用PyTorch实现LSTNet模型的代码示例,其中包括了LSTM层、卷积层和外部记忆模块的实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class LSTNet(nn.Module):
def __init__(self, input_size, hidden_size, output_size, kernel_size, num_layers, dilation, dropout):
"""
LSTNet模型初始化函数
:param input_size: 输入数据的特征维度
:param hidden_size: LSTM的隐藏层维度
:param output_size: 输出数据的特征维度
:param kernel_size: 卷积层的卷积核大小
:param num_layers: LSTM的层数
:param dilation: 卷积层的膨胀因子
:param dropout: Dropout概率
"""
super(LSTNet, self).__init__()
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
self.kernel_size = kernel_size
self.num_layers = num_layers
self.dilation = dilation
self.dropout = dropout
# 卷积层
self.conv = nn.Conv2d(1, hidden_size, kernel_size=(kernel_size, 1), stride=(1, 1),
padding=((kernel_size-1)*dilation, 0), dilation=(dilation, 1))
# LSTM层
self.lstm = nn.LSTM(input_size=hidden_size, hidden_size=hidden_size, num_layers=num_layers, dropout=dropout)
# 外部记忆模块
self.gate = nn.Linear(hidden_size, hidden_size)
self.memory = nn.Linear(hidden_size, hidden_size)
self.out = nn.Linear(hidden_size, output_size)
def forward(self, x):
"""
LSTNet模型前向传播函数
:param x: 输入数据,形状为(batch_size, input_size, seq_len)
:return: 输出数据,形状为(batch_size, output_size)
"""
# 卷积层特征提取
x = F.pad(x.unsqueeze(1), (self.kernel_size-1)*self.dilation, 0)
x = self.conv(x).squeeze(-1)
x = F.relu(x)
# LSTM层处理
x = x.permute(2, 0, 1)
h = torch.zeros(self.num_layers, x.shape[1], self.hidden_size).to(x.device)
c = torch.zeros(self.num_layers, x.shape[1], self.hidden_size).to(x.device)
for i in range(x.shape[0]):
output, (h, c) = self.lstm(x[i].unsqueeze(0), (h, c))
if i == 0:
outputs = output
else:
outputs = torch.cat([outputs, output], dim=0)
outputs = F.dropout(outputs, p=self.dropout, training=self.training)
# 外部记忆模块
gates = torch.sigmoid(self.gate(outputs))
memory = F.relu(self.memory(outputs))
outputs = torch.mul(gates, memory).sum(dim=0)
# 输出层
outputs = self.out(outputs)
return outputs.squeeze(-1)
```
在这个实现中,我们使用了PyTorch中的nn.Module类来定义LSTNet模型,并分别实现了卷积层、LSTM层和外部记忆模块。在前向传播函数中,我们首先使用卷积层对输入数据进行特征提取,然后将特征映射到LSTM层中进行处理,并使用外部记忆模块来学习序列中的周期性模式。最后,我们使用一个输出层将LSTM层的输出映射到输出数据的特征维度上。
相关推荐
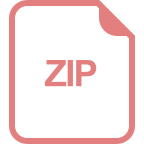
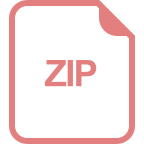
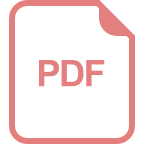














