C语言求二叉树以元素值为x的节点为根的子树深度代码
时间: 2023-06-06 19:06:53 浏览: 94
以下是C语言求二叉树以元素值为x的节点为根的子树深度的代码实现:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
int subtreeDepth(TreeNode* root, int x) {
if (root == NULL) {
return 0;
}
if (root->val == x) {
return maxDepth(root);
}
int leftDepth = subtreeDepth(root->left, x);
int rightDepth = subtreeDepth(root->right, x);
return leftDepth > rightDepth ? leftDepth : rightDepth;
}
int maxDepth(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
int main() {
// 构建二叉树
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = 1;
root->left = (TreeNode*)malloc(sizeof(TreeNode));
root->left->val = 2;
root->left->left = NULL;
root->left->right = NULL;
root->right = (TreeNode*)malloc(sizeof(TreeNode));
root->right->val = 3;
root->right->left = (TreeNode*)malloc(sizeof(TreeNode));
root->right->left->val = 4;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right = NULL;
int x = 3;
int depth = subtreeDepth(root, x);
printf("Subtree depth for node with val %d is %d\n", x, depth);
return 0;
}
```
该代码实现了一个查找二叉树中以元素值为x的节点为根的子树深度的函数subtreeDepth,并且使用了maxDepth函数来计算最大深度。如果节点查找失败或者树为空,subtreeDepth函数会返回0。
阅读全文
相关推荐
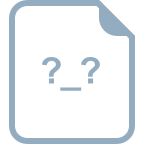

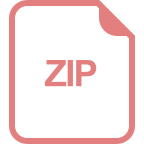



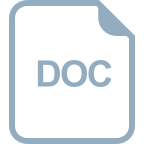
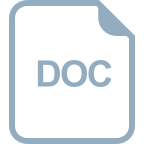








