用java语言回溯法编写一个实验程序,求出a、b、c、d、e,满足ab-cd+e-1方程,其中所有变量的取值为1~5并且均不相同。
时间: 2024-01-25 22:04:07 浏览: 83
以下是使用Java语言编写的回溯法程序,用于求解方程ab-cd+e-1=0的解,其中变量a、b、c、d、e均为1~5之间的整数且不相同。
```java
public class EquationSolver {
private static final int N_VARIABLES = 5;
private static final int[] VALUES = {1, 2, 3, 4, 5};
private int[] solution; // 保存当前解
public EquationSolver() {
solution = new int[N_VARIABLES];
}
public void solve() {
backtrack(0);
}
private void backtrack(int variableIndex) {
if (variableIndex == N_VARIABLES) { // 找到一个解
int a = solution[0];
int b = solution[1];
int c = solution[2];
int d = solution[3];
int e = solution[4];
if (a * b - c * d + e - 1 == 0) {
System.out.printf("%d * %d - %d * %d + %d - 1 = 0\n", a, b, c, d, e);
}
return;
}
for (int i = 0; i < VALUES.length; i++) {
if (isValid(variableIndex, VALUES[i])) {
solution[variableIndex] = VALUES[i];
backtrack(variableIndex + 1);
solution[variableIndex] = 0; // 回溯
}
}
}
private boolean isValid(int variableIndex, int value) {
// 检查当前变量的值是否与之前的变量的值相同
for (int i = 0; i < variableIndex; i++) {
if (solution[i] == value) {
return false;
}
}
return true;
}
public static void main(String[] args) {
EquationSolver solver = new EquationSolver();
solver.solve();
}
}
```
该程序使用回溯法求解方程的解,从变量a开始逐个尝试取1~5之间的值,再依次尝试变量b、c、d、e的取值,直到找到一个满足方程的解或者所有的变量都尝试完毕。在回溯的过程中,需要保证每个变量的取值都不与之前的变量取值相同。如果找到一个解,则输出该解的值。
阅读全文
相关推荐











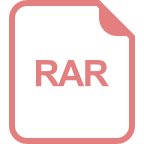
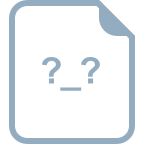
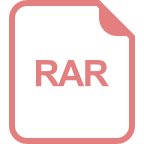