istringstream 的构造函数没有接受 string 类型的参数,因此无法实例化对象
时间: 2023-05-24 19:07:11 浏览: 56
。正确的做法是使用一个字符串作为输入流的参数,并将其传递给构造函数。例如:
```cpp
#include <iostream>
#include <sstream>
#include <string>
using namespace std;
int main() {
string s = "hello world";
istringstream iss(s);
string word;
while (iss >> word) {
cout << word << endl;
}
return 0;
}
```
这里使用一个字符串 s 创建了一个输入流对象 iss,并使用 while 循环读取流中的单词并输出。
相关问题
istringstream函数
istringstream函数是C++中的一个类,用于从字符串中读取字符。其构造函数的原型为istringstream::istringstream(string str),其中str为待读取的字符串。通过使用该函数,可以逐个读取字符串中的字符,并进行相应的处理操作。
示例代码如下:
```
#include<iostream>
#include<sstream>
#include<string>
using namespace std;
int main() {
string str = "this is mine";
istringstream is(str);
string s;
while (is >> s)
cout << s << endl;
return 0;
}
```
以上示例中,我们创建了一个istringstream对象is,并将待读取的字符串str作为参数传入其构造函数中。然后,通过while循环以空格为分隔符,逐个读取字符串中的单词,并将其输出到屏幕上。
编写两个函数模板,实现将各种数据类和string类型互换型
以下是两个函数模板的实现:
```cpp
#include <iostream>
#include <string>
template<typename T>
std::string to_string(T value) {
return std::to_string(value);
}
template<>
std::string to_string<std::string>(std::string value) {
return value;
}
template<typename T>
T from_string(std::string str) {
T value;
std::istringstream iss(str);
iss >> value;
return value;
}
template<>
std::string from_string<std::string>(std::string str) {
return str;
}
int main() {
int x = 123;
std::string str = to_string(x);
std::cout << str << std::endl;
double y = 3.14;
str = to_string(y);
std::cout << str << std::endl;
std::string s = "hello";
str = to_string(s);
std::cout << str << std::endl;
int a = from_string<int>("456");
std::cout << a << std::endl;
double b = from_string<double>("6.28");
std::cout << b << std::endl;
std::string c = from_string<std::string>("world");
std::cout << c << std::endl;
return 0;
}
```
第一个函数模板 `to_string` 实现了将各种数据类型转换为字符串类型。如果传入的是 `std::string` 类型,则直接返回原字符串。
第二个函数模板 `from_string` 实现了将字符串类型转换为各种数据类型。如果传入的是 `std::string` 类型,则直接返回原字符串。
在 `main` 函数中,我们测试了将整型、浮点型和字符串类型转换为字符串类型,以及将字符串类型转换为整型、浮点型和字符串类型的功能。
相关推荐
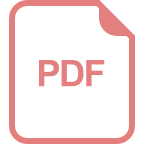












