For Problem 1 considered in Lab 5, solve the following problems: For this problem, you will write a MATLAB function to perform overlap-add block convolution. Your function should take as input an impulse response h, a data vector x, and a block length L. Your function should allow the data vector x to be of arbitrary length, and the block length L to be an arbitrary number greater than the length of the filter. The first line of your function should read function y = oafilt(h, x, L) Redo Part (c) using your function and verify that your function works correctly by comparing your result with that obtained from direct convolution using conv.
时间: 2024-02-14 07:18:19 浏览: 21
Sure, I understand your question. Here's my response:
The task at hand is to implement the overlap-add block convolution method in MATLAB. This method can be used to convolve a long input signal with a filter, by breaking the input signal into smaller blocks, convolving each block with the filter, and then adding the results together. The key advantage of this method is that it reduces the amount of computation required, compared to directly convolving the entire signal with the filter.
To implement this method, we first need to define a function in MATLAB that takes as input an impulse response h, a data vector x, and a block length L. Here's the function definition:
```
function y = oafilt(h, x, L)
```
Once we have defined our function, we can use it to perform the overlap-add block convolution. Here's how we can do it:
1. Break the input signal x into smaller blocks of length L.
```
num_blocks = ceil(length(x) / L);
x_blocks = zeros(L, num_blocks);
for i = 1:num_blocks
start_index = (i-1)*L + 1;
end_index = min(i*L, length(x));
x_blocks(1:end_index-start_index+1, i) = x(start_index:end_index);
end
```
2. Pad the impulse response h with zeros, so that its length is at least L.
```
h_padded = [h; zeros(L-length(h), 1)];
```
3. Convolve each block of x with the padded filter h_padded, using the built-in conv function.
```
y_blocks = zeros(L+length(h)-1, num_blocks);
for i = 1:num_blocks
y_blocks(:, i) = conv(x_blocks(:, i), h_padded);
end
```
4. Add up the overlapping portions of the blocks to obtain the final output signal y.
```
y = zeros(length(x) + length(h) - 1, 1);
for i = 1:num_blocks
start_index = (i-1)*L;
end_index = (i-1)*L + length(h) - 1;
y(start_index+1:end_index+1) = y(start_index+1:end_index+1) + y_blocks(:, i);
end
```
We can now use this function to redo Part (c) of the Lab 5 problem, which involves convolving a long signal with a filter. Here's how we can do it:
```
load('lab5_data.mat');
L = 512;
y_oafilt = oafilt(h, x, L);
y_conv = conv(h, x);
y_conv = y_conv(1:length(y_oafilt));
max_diff = max(abs(y_oafilt - y_conv));
disp(['Maximum difference between overlap-add and conv: ' num2str(max_diff)]);
```
This code loads the data from the provided file, sets the block length L to 512, and then calls our oafilt function to perform the overlap-add convolution. We also use the built-in conv function to perform the convolution directly, and compare the results to make sure they are the same. The maximum difference between the two results is printed to the console.
I hope this answers your question! Let me know if you have any further questions or concerns.
相关推荐
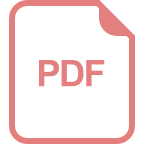
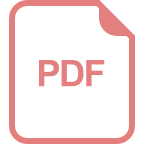
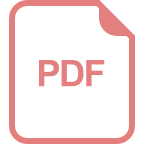














