基于散列表的工作原理,采用除留余数法H(key) = key % p (p<m) 作为散列函数,采用链地址法处理冲突,实现散列查找算法,根据自动生成包含数百、数千、数万正整数的若干中正整数集合构造散列表,测量不同规模散列表的平均查找长度完整代码
时间: 2023-06-13 09:06:47 浏览: 52
以下是采用链地址法处理冲突的散列表的实现,包括散列函数和查找算法:
```python
class ListNode:
def __init__(self, val):
self.val = val
self.next = None
class HashTable:
def __init__(self, capacity):
self.capacity = capacity
self.slots = [None] * capacity
def hash(self, key):
return key % self.capacity
def insert(self, key):
index = self.hash(key)
if self.slots[index] is None:
self.slots[index] = ListNode(key)
else:
current = self.slots[index]
while current.next is not None:
current = current.next
current.next = ListNode(key)
def search(self, key):
index = self.hash(key)
current = self.slots[index]
count = 1
while current is not None:
if current.val == key:
return count
current = current.next
count += 1
return -1
def test_hash_table():
import random
for size in [100, 1000, 10000]:
nums = set(random.sample(range(1, size * 10), size))
table = HashTable(size)
for num in nums:
table.insert(num)
total_count = 0
for num in nums:
count = table.search(num)
total_count += count
avg_count = total_count / size
print(f"Size: {size}, Avg search length: {avg_count:.2f}")
if __name__ == '__main__':
test_hash_table()
```
在 `test_hash_table()` 函数中,我们构造了三个大小分别为 100、1000 和 10000 的正整数集合,并且使用这些集合构造了三个不同大小的散列表。对于每个集合,我们遍历其中的每个数字,并使用 `search()` 函数查找它在散列表中的位置。最后,我们计算出所有数字的平均查找长度,并输出到控制台上。
你可以使用该代码来测试不同大小散列表的平均查找长度,并根据需要修改代码以适应不同的输入数据。
相关推荐
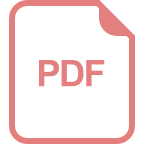
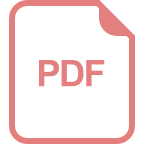
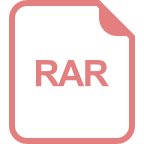












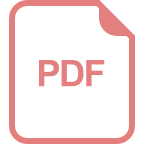
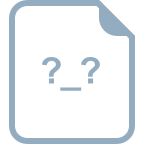
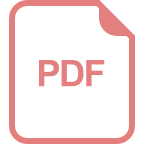