Description: Write a program that prints a right-angled triangle pattern of stars using nested loops
时间: 2024-10-26 07:16:20 浏览: 18
创建一个右三角形模式的程序,我们可以利用嵌套循环来实现。这里有两个例子展示了不同的布局策略:
1. **基于元素边距**[^1]:如果我们想要元素左对齐到父元素右侧,可以这样设计一个三角形,每个星号代表一行:
```plaintext
*
* *
* * *
```
在这个例子中,我们可能不会直接打印星号形成三角形,但可以通过控制文本元素的宽度和位置来模拟。
2. **填充整个父元素**:另一种方式是让元素充满其父元素,然后通过调整内层元素的位置来构建三角形,比如这样:
```plaintext
*
**
***
****
```
这通常是通过设置元素的`anchors.fill`属性来实现满屏显示,然后调整内部元素的间距。
要编写一个具体的程序,我们可以选择任意一种方法,具体取决于你的布局需求。例如,使用Python控制字符输出(假设输出是控制台):
```python
for i in range(1, 5): # 从1开始以避免空行
for j in range(i):
print("*", end="")
print()
```
这个简单的Python脚本会打印一个标准的右三角形。
相关问题
Write a program that takes a list of strings and prints the length of each string using a for loop.
To write a program in C++ that takes a list of strings and prints the length of each string using a `for` loop, you would first need to declare an array or vector of strings. Here's a simple example:
```cpp
#include <iostream>
#include <vector> // Include the library for dynamic arrays
int main() {
std::vector<std::string> stringList = {"apple", "banana", "cherry"}; // Declare a vector of strings
// Iterate through the vector using a for loop
for (const auto& str : stringList) { // Using range-based for loop for simplicity
std::cout << "Length of string \"" << str << "\": " << str.length() << std::endl;
}
return 0;
}
```
In this code snippet, we use the `std::vector` container from `<vector>` to store the strings. The `for` loop iterates over each string (`str`) in the vector, and we call the `length()` method to get the length of the string. The result is then printed to the console.
Write a Python program that asks the user to input a number and prints a Eiffel Tower of stars based on that number.
当然可以。首先,我们需要理解题目的需求,即创建一个Python程序,用户输入一个数字,然后打印出对应数量的星字符来模拟埃菲尔铁塔的样子。这里我们假设埃菲尔铁塔是通过每一层逐渐减小星星的数量来构建的。
下面是一个简单的示例,使用嵌套循环来实现这个功能:
```python
# 获取用户输入的数字
num_stars = int(input("请输入一个数字,将以此生成埃菲尔铁塔形状的星号图案:"))
# 设置每层星星的宽度
max_width = num_stars
# 初始化层数和当前层的星星数
level = 0
stars_per_row = max_width
for i in range(num_stars * 2): # 埃菲尔铁塔通常有三层,所以乘以2
if level == 0 or level == 2:
print(' ' * (max_width - stars_per_row), end='') # 对齐空白字符
else:
print(' ' * (max_width // 2), '*' * stars_per_row, ' ' * (max_width // 2)) # 层间空隙
stars_per_row -= 2 # 每层减少星星数
level += 1
print() # 换行开始下一层
阅读全文
相关推荐
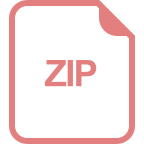
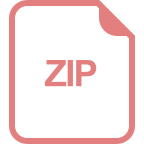
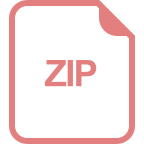













