C++,Write a program that prints out the weekly pay summary based on user input. Your program should include the following global functions contained in a namespace called “employeePayment” void askValue(string, char&) void askValue(string, string&) vo
时间: 2024-01-07 16:03:36 浏览: 28
Here is an example program in C++ that uses the `employeePayment` namespace to prompt the user for input and calculate weekly pay:
```cpp
#include <iostream>
#include <iomanip>
#include <string>
namespace employeePayment {
void askValue(std::string prompt, char& value) {
std::cout << prompt;
std::cin >> value;
}
void askValue(std::string prompt, std::string& value) {
std::cout << prompt;
std::cin >> value;
}
double calculatePay(double hoursWorked, double hourlyRate) {
double regularPay = 0.0;
double overtimePay = 0.0;
if (hoursWorked <= 40.0) {
regularPay = hoursWorked * hourlyRate;
}
else {
regularPay = 40.0 * hourlyRate;
overtimePay = (hoursWorked - 40.0) * (hourlyRate * 1.5);
}
return regularPay + overtimePay;
}
}
int main() {
double hoursWorked;
double hourlyRate;
std::string name;
char employeeType;
employeePayment::askValue("Enter employee name: ", name);
employeePayment::askValue("Enter employee type (H or S): ", employeeType);
employeePayment::askValue("Enter hours worked: ", hoursWorked);
employeePayment::askValue("Enter hourly rate: ", hourlyRate);
double weeklyPay = employeePayment::calculatePay(hoursWorked, hourlyRate);
std::cout << std::fixed << std::setprecision(2);
std::cout << "Weekly pay summary for " << name << std::endl;
std::cout << "Employee type: " << employeeType << std::endl;
std::cout << "Hours worked: " << hoursWorked << std::endl;
std::cout << "Hourly rate: $" << hourlyRate << std::endl;
std::cout << "Regular pay: $" << (hoursWorked <= 40.0 ? weeklyPay : 40.0 * hourlyRate) << std::endl;
std::cout << "Overtime pay: $" << (hoursWorked > 40.0 ? (hoursWorked - 40.0) * (hourlyRate * 1.5) : 0.0) << std::endl;
std::cout << "Total pay: $" << weeklyPay << std::endl;
return 0;
}
```
This program prompts the user for the employee's name, type (whether they are hourly or salaried), hours worked, and hourly rate. It then calculates the employee's weekly pay based on this information and prints out a summary of the results.
The `employeePayment` namespace contains two functions for prompting the user for input: `askValue(std::string, char&)` and `askValue(std::string, std::string&)`. These functions are used to get the user's input for the employee's name and type.
The `calculatePay(double, double)` function takes in the hours worked and hourly rate and calculates the employee's weekly pay. If the employee worked more than 40 hours, overtime pay is also calculated.
In `main()`, the program calls the `askValue()` functions to get the user's input, then calls `calculatePay()` to calculate the employee's weekly pay. Finally, it prints out a summary of the results using `std::cout`.
相关推荐
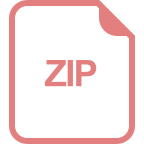
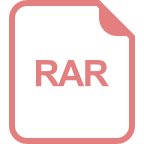
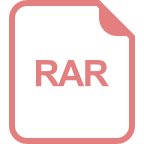















