Write a loop that prints a triangle as shown below. * ** *** **** ***** **** *** ** *
时间: 2023-07-01 10:25:11 浏览: 29
以下是使用Python编写循环打印三角形的代码:
```
for i in range(1, 6): # 控制行数
for j in range(i): # 控制每行的列数
print('*', end='')
print()
for i in range(4, 0, -1): # 控制行数
for j in range(i): # 控制每行的列数
print('*', end='')
print()
```
在上面的代码中,我们使用两个for循环来控制三角形的行数和每行的列数。第一个循环从1到5,每次打印一行星号,第二个循环从4到1,每次打印一行星号。注意,我们使用了end=''来确保星号不换行。
相关问题
void enqueue(SeqQueue *q, ElemType item)
As an AI language model, I cannot infer the exact implementation details of your code. However, I can provide you with a general idea of how the `enqueue` function for a sequential queue might work.
The `enqueue` function is used to add an element to the back of the queue. In other words, it inserts the new element after the last element in the queue. Here is one possible implementation in C:
```
void enqueue(SeqQueue *q, ElemType item) {
// Check if the queue is full
if (q->rear == MAX_SIZE - 1) {
printf("Queue is full\n");
return;
}
// Increment the rear index and add the new item
q->rear++;
q->data[q->rear] = item;
printf("%d enqueued to queue\n", item);
}
```
This implementation assumes that the sequential queue data structure has the following properties:
- `MAX_SIZE`: the maximum number of elements the queue can hold
- `SeqQueue`: a struct that contains the queue's front and rear indices, as well as an array to hold the queue elements (`data`)
- `ElemType`: the data type of the queue elements
The `enqueue` function first checks if the queue is full (i.e., if the rear index is equal to `MAX_SIZE - 1`). If so, it prints an error message and returns without adding the new element.
Otherwise, it increments the rear index and adds the new element to the `data` array at the index indicated by the new rear index. Finally, it prints a message indicating that the element was successfully enqueued.
make: *** [amqp_channel.lo] Error 1 ERROR: `make' failed
As an AI language model, I cannot know the specific reason why `make` failed in your case. However, here are some general troubleshooting steps you can take:
1. Check if all the necessary dependencies are installed: `make` may fail if required dependencies are missing. Check the documentation or installation instructions for the software you are trying to build and ensure that all dependencies are installed.
2. Look for error messages: `make` often prints out error messages when it fails. Look for these messages in the terminal output to get a better understanding of what went wrong.
3. Check the logs: Sometimes, the error message may not provide enough information to troubleshoot the issue. In such cases, check the logs generated by `make` to get more information.
4. Try building in a clean environment: A clean environment may help to identify the cause of the error. Try building the software in a fresh environment, such as a new virtual machine or container.
5. Seek help: If all else fails, seek help from the software's community, such as forums or mailing lists. Other users may have faced similar issues and may be able to provide guidance on how to resolve the issue.
相关推荐
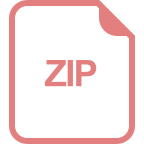
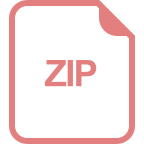
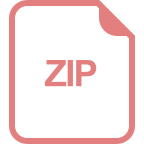












