帮我用python代码实现以下功能:批量读取所有txt文件,每行第一个数字为2的保留,其余行都删除
时间: 2023-12-27 15:04:09 浏览: 23
可以使用以下代码实现:
```python
import os
# 遍历文件夹中的所有txt文件
for filename in os.listdir('.'):
if filename.endswith('.txt'):
with open(filename, 'r') as f:
# 读取文件中的每一行
lines = f.readlines()
with open(filename, 'w') as f:
# 重新写入文件,只保留第一个数字为2的行
for line in lines:
if line.strip().startswith('2'):
f.write(line)
```
这段代码首先使用 `os.listdir()` 函数遍历当前文件夹中的所有文件,然后筛选出以 `.txt` 结尾的文件。对于每个符合条件的文件,使用 `open()` 函数读取每一行内容,然后再次使用 `open()` 函数以写入模式打开文件,只保留第一个数字为2的行重新写入文件中。
相关问题
帮我用python代码实现以下功能:批量读取所有txt文件,每行第一个数字为2的保留,其余行都删除,如果某一个txt文件第一列没有数字2,则删除这个txt文件
好的,以下是实现功能的代码:
```python
import os
# 定义要读取的txt文件所在的文件夹路径
folder_path = './txt_files/'
# 遍历文件夹中的所有txt文件
for file_name in os.listdir(folder_path):
# 判断文件名是否以.txt结尾
if file_name.endswith('.txt'):
with open(os.path.join(folder_path, file_name), 'r', encoding='utf-8') as f:
# 读取txt文件中的所有行
lines = f.readlines()
# 判断第一列是否有数字2
if '2' not in lines[0].split()[0]:
# 如果没有,则删除文件
os.remove(os.path.join(folder_path, file_name))
else:
# 如果有,保留第一行数字为2的行,删除其他行
with open(os.path.join(folder_path, file_name), 'w', encoding='utf-8') as f:
f.write(lines[0])
for line in lines[1:]:
if line.startswith('2'):
f.write(line)
```
需要注意的是,代码中的`./txt_files/`是指txt文件所在的文件夹路径,需要根据实际情况进行修改。另外,代码中的编码方式是`utf-8`,如果实际情况中txt文件的编码方式不同,需要进行相应的修改。
帮我用python写一段代码实现以下功能:批量打开txt文件,并把每个txt文件中第一列的数字修改为指定的数字
好的,可以按照以下代码进行实现:
```python
import os
def modify_first_column(directory, target_number):
for filename in os.listdir(directory):
if filename.endswith(".txt"):
filepath = os.path.join(directory, filename)
with open(filepath, "r") as f:
lines = f.readlines()
for i in range(len(lines)):
line = lines[i].strip().split()
try:
line[0] = str(target_number)
except IndexError:
continue
lines[i] = " ".join(line) + "\n"
with open(filepath, "w") as f:
f.writelines(lines)
directory = "path/to/txt/files"
target_number = 123
modify_first_column(directory, target_number)
```
其中,`directory` 是包含 txt 文件的目录路径,`target_number` 是要替换成的数字。函数 `modify_first_column` 循环遍历目录中的每个 txt 文件,打开文件并读取每行内容,将每行按空格分割成列表,将第一个元素替换为 `target_number`,然后再将列表转换为字符串并写回文件中。最终,所有 txt 文件中的第一列数字都被替换为 `target_number`。
相关推荐
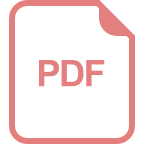
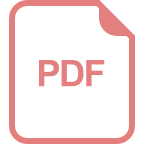












