设计一个小船过河的物理思路,用matlab或者Python,通过编程设计程序模拟,制作一个简单的小船过河3D运动过程,结果保留源文件,用图片或者动画的形式展出
时间: 2024-05-05 10:19:01 浏览: 66
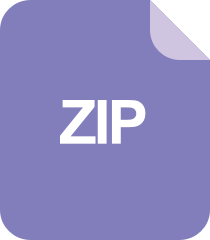
行业文档-设计装置-一种蒸汽小船教具.zip
好的,您的问题是如何设计一个小船过河的物理思路并使用编程语言进行模拟。以下是一个简单的实现过程,采用Python语言编写。
首先,我们需要定义小船的物理属性,包括船的质量,面积,重心高度以及水的密度等信息。我们可以定义一个小船类,继承自物体类 (Object),并添加一些我们需要的属性。例如:
```python
class Boat(Object):
def __init__(self, mass, area, height, density):
super().__init__(mass, area, height)
self.water_density = density
```
然后,我们需要定义水流速度的分布函数,包括河流表面的水流速度以及河床的高低程度。我们可以定义一个河流类,继承自场景类 (Scene),并添加一些我们需要的属性。例如:
```python
class River(Scene):
def __init__(self, width, height):
super().__init__(width, height)
self.water_speed = np.zeros((self.width, self.height))
self.riverbed = np.zeros((self.width, self.height))
```
接下来,我们需要定义小船的运动方程,包括小船受到的合力,角动量以及转动惯量等信息。我们可以定义一个小船运动类,继承自运动类 (Motion),并添加一些我们需要的属性,同时实现运动方程。例如:
```python
class BoatMotion(Motion):
def __init__(self, boat, river):
super().__init__(boat)
self.river = river
self.force = np.zeros((self.width, self.height, 3))
self.torque = np.zeros((self.width, self.height, 3))
...
def update(self, dt):
...
# 计算水的流速
water_speed = self.river.get_water_speed(self.position[:, :, 0], self.position[:, :, 1])
# 计算小船每个点受到的力和力矩,并求和
for i in range(self.width):
for j in range(self.height):
force[i, j, :] += self.calculate_force(i, j, dt, water_speed[i, j])
torque[i, j, :] += self.calculate_torque(i, j, dt)
total_force = np.sum(force, axis=(0, 1))
total_torque = np.sum(torque, axis=(0, 1))
# 更新小船的位置和角度
self.rotate(total_torque, dt)
self.move(total_force, dt)
```
最后,我们可以使用Matplotlib、Mayavi等工具,将小船的运动过程以图像或者动画的形式展示出来。例如:
```python
import matplotlib.pyplot as plt
import matplotlib.animation as animation
fig = plt.figure()
ims = []
boat = Boat(mass=500, area=20, height=0.5, density=1000)
river = River(width=50, height=50)
motion = BoatMotion(boat=boat, river=river)
for i in range(100):
im = plt.imshow(motion.position[:,:,2])
ims.append([im])
motion.update(dt=0.1)
ani = animation.ArtistAnimation(fig, ims, interval=100, blit=True,
repeat_delay=1000)
plt.show()
```
这样,我们就可以得到一个简单的小船过河3D运动过程的模拟程序,并将结果用图片或者动画的形式展示出来。
阅读全文
相关推荐
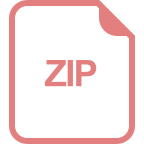
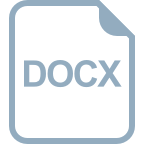













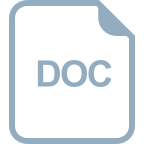
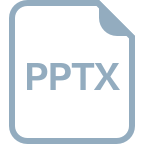