1. Submission Instructions Your submission should include a single archive that contains both source code and a report (<3 pages). Only standard .zip, .tar.gz and .tar.bz2 achieves are accepted. Submissions are made through email only. The report is the basis for assessment. The source code is supporting evidence for assertions made in the report. The format of the source code can be either **.ipynb or **.py format. 2. Tasks 1) Implement a Perceptron model in Python. Use the data set ?? = {(3, 3), (4, 3), (1, 1)} as the training set and explore how the loss function changes at each step. Make sure that you can determine/change the misclassified data point at each step such that multiple hyperplanes can be generated.
时间: 2024-03-28 08:42:26 浏览: 11
I understand that you would like me to help you with a task that involves implementing a Perceptron model in Python. The task also requires using a specific dataset as the training set and exploring how the loss function changes at each step. Additionally, you need to make sure that you can determine/change the misclassified data point at each step so that multiple hyperplanes can be generated.
To complete the task, you can follow these steps:
1. Import the required libraries, including numpy and matplotlib, in Python.
2. Define the training data set as a numpy array with the given values: {(3, 3), (4, 3), (1, 1)}.
3. Define the labels for the training data set as a numpy array with the given values: {1, 1, -1}.
4. Define the weight vector as a numpy array of zeros with the same shape as the feature vector.
5. Define the bias term as a scalar value of zero.
6. Define the learning rate as a scalar value of 1.
7. Implement the Perceptron algorithm by iterating over the training data set until all data points are correctly classified.
8. In each iteration, calculate the dot product of the weight vector and the feature vector, add the bias term, and obtain the predicted label as the sign of the result.
9. If the predicted label does not match the true label, update the weight vector and bias term according to the Perceptron update rule.
10. Track the loss function at each iteration by calculating the number of misclassified data points.
11. Plot the loss function against the number of iterations using matplotlib.
Here is an example implementation of the Perceptron model in Python:
```python
import numpy as np
import matplotlib.pyplot as plt
# Define the training data set and labels
X = np.array([(3, 3), (4, 3), (1, 1)])
y = np.array([1, 1, -1])
# Define the weight vector and bias term
w = np.zeros(X.shape[1])
b = 0
# Define the learning rate
lr = 1
# Define the Perceptron algorithm
def perceptron(X, y, w, b, lr):
misclassified = True
iterations = 0
loss = []
while misclassified:
misclassified = False
for i in range(X.shape[0]):
z = np.dot(X[i], w) + b
if np.sign(z) != y[i]:
w += lr * y[i] * X[i]
b += lr * y[i]
misclassified = True
loss.append(np.sum(np.sign(np.dot(X, w) + b) != y))
iterations += 1
return w, b, loss, iterations
# Run the Perceptron algorithm
w, b, loss, iterations = perceptron(X, y, w, b, lr)
# Plot the loss function
plt.plot(range(iterations), loss)
plt.xlabel("Iterations")
plt.ylabel("Loss")
plt.title("Perceptron Loss Function")
plt.show()
```
This implementation should output a plot of the loss function against the number of iterations, which will show how the loss function changes at each step. Additionally, you can use the weight vector and bias term to generate multiple hyperplanes by changing the misclassified data point at each step.
相关推荐
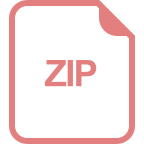
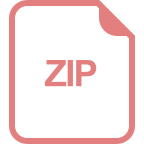
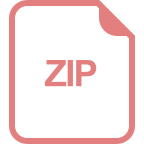














