通过设计程序,实现磁盘寻道算法中FCFS、SSTF、SCAN和CSCAN四种算法C++
时间: 2024-02-05 20:05:18 浏览: 148
好的,以下是四种磁盘寻道算法的C++程序实现。
1. 先来先服务算法(FCFS)
```c++
#include <iostream>
#include <cmath>
using namespace std;
int FCFS(int disk_queue[], int size, int start_pos) {
int total_distance = 0;
int current_pos = start_pos;
for (int i = 0; i < size; i++) {
int distance = abs(disk_queue[i] - current_pos);
total_distance += distance;
current_pos = disk_queue[i];
}
return total_distance;
}
int main() {
int disk_queue[] = {98, 183, 37, 122, 14, 124, 65, 67};
int size = sizeof(disk_queue) / sizeof(disk_queue[0]);
int start_pos = 53;
int total_distance = FCFS(disk_queue, size, start_pos);
cout << "FCFS: " << total_distance << endl;
return 0;
}
```
2. 最短寻道时间优先算法(SSTF)
```c++
#include <iostream>
#include <cmath>
using namespace std;
int SSTF(int disk_queue[], int size, int start_pos) {
int total_distance = 0;
int current_pos = start_pos;
while (size > 0) {
int min_distance = INT_MAX;
int next_pos = 0;
for (int i = 0; i < size; i++) {
int distance = abs(disk_queue[i] - current_pos);
if (distance < min_distance) {
min_distance = distance;
next_pos = i;
}
}
total_distance += min_distance;
current_pos = disk_queue[next_pos];
for (int i = next_pos; i < size - 1; i++) {
disk_queue[i] = disk_queue[i+1];
}
size--;
}
return total_distance;
}
int main() {
int disk_queue[] = {98, 183, 37, 122, 14, 124, 65, 67};
int size = sizeof(disk_queue) / sizeof(disk_queue[0]);
int start_pos = 53;
int total_distance = SSTF(disk_queue, size, start_pos);
cout << "SSTF: " << total_distance << endl;
return 0;
}
```
3. 扫描算法(SCAN)
```c++
#include <iostream>
#include <cmath>
#include <algorithm>
using namespace std;
int SCAN(int disk_queue[], int size, int start_pos) {
int total_distance = 0;
int current_pos = start_pos;
sort(disk_queue, disk_queue + size);
int index = 0;
for (int i = 0; i < size; i++) {
if (disk_queue[i] > start_pos) {
index = i;
break;
}
}
bool direction = true;
while (index >= 0 && index < size) {
if (direction) {
total_distance += abs(disk_queue[index] - current_pos);
current_pos = disk_queue[index];
index++;
if (index == size) {
total_distance += abs(current_pos - 199);
current_pos = 199;
direction = false;
index--;
}
} else {
total_distance += abs(disk_queue[index] - current_pos);
current_pos = disk_queue[index];
index--;
if (index < 0) {
total_distance += abs(current_pos - 0);
current_pos = 0;
direction = true;
index = 0;
}
}
}
return total_distance;
}
int main() {
int disk_queue[] = {98, 183, 37, 122, 14, 124, 65, 67};
int size = sizeof(disk_queue) / sizeof(disk_queue[0]);
int start_pos = 53;
int total_distance = SCAN(disk_queue, size, start_pos);
cout << "SCAN: " << total_distance << endl;
return 0;
}
```
4. 循环扫描算法(CSCAN)
```c++
#include <iostream>
#include <cmath>
#include <algorithm>
using namespace std;
int CSCAN(int disk_queue[], int size, int start_pos) {
int total_distance = 0;
int current_pos = start_pos;
sort(disk_queue, disk_queue + size);
int index = 0;
for (int i = 0; i < size; i++) {
if (disk_queue[i] > start_pos) {
index = i;
break;
}
}
bool direction = true;
while (index >= 0 && index < size) {
if (direction) {
total_distance += abs(disk_queue[index] - current_pos);
current_pos = disk_queue[index];
index++;
if (index == size) {
total_distance += abs(current_pos - 199);
current_pos = 199;
direction = false;
index = 0;
}
} else {
total_distance += abs(disk_queue[index] - current_pos);
current_pos = disk_queue[index];
index--;
if (index < 0) {
total_distance += abs(current_pos - 0);
current_pos = 0;
direction = true;
index = 0;
}
}
}
return total_distance;
}
int main() {
int disk_queue[] = {98, 183, 37, 122, 14, 124, 65, 67};
int size = sizeof(disk_queue) / sizeof(disk_queue[0]);
int start_pos = 53;
int total_distance = CSCAN(disk_queue, size, start_pos);
cout << "CSCAN: " << total_distance << endl;
return 0;
}
```
以上四种磁盘寻道算法的程序实现均为C++语言。
阅读全文
相关推荐
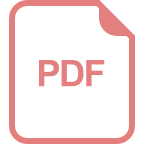


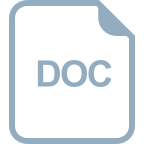
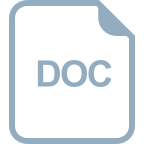
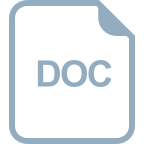
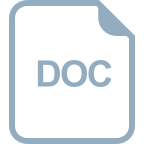
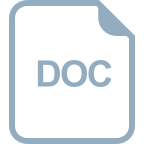
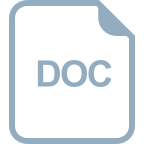







