使用C语言写出3个ADC读取程序
时间: 2023-09-11 09:04:39 浏览: 52
### 回答1:
很高兴为您回答。以下是三个使用 C 语言编写的 ADC 读取程序:
1. 简单读取程序:
```
#include <stdio.h>
int main(void)
{
int adc_value;
// 在这里,请使用相应的ADC驱动代码读取ADC数值
printf("ADC Value: %d\n", adc_value);
return 0;
}
```
2. 读取并打印连续ADC数值的程序:
```
#include <stdio.h>
int main(void)
{
int adc_value;
int i;
for (i = 0; i < 10; i++) {
// 在这里,请使用相应的ADC驱动代码读取ADC数值
printf("ADC Value [%d]: %d\n", i, adc_value);
}
return 0;
}
```
3. 读取并计算平均ADC数值的程序:
```
#include <stdio.h>
int main(void)
{
int adc_value;
int i;
int sum = 0;
for (i = 0; i < 10; i++) {
// 在这里,请使用相应的ADC驱动代码读取ADC数值
sum += adc_value;
}
printf("ADC Average Value: %d\n", sum / 10);
return 0;
}
```
希望这些代码可以帮助您开始编写您的 ADC 读取程序。
### 回答2:
使用C语言编写3个ADC读取程序的例子:
第一个程序:
```c
#include <stdio.h>
int main() {
int adcValue;
// 假设ADC引脚为A0
// 初始化ADC模块
// ...
// 开始读取ADC值
adcValue = readADC(0); // 读取A0引脚的ADC值
printf("ADC值: %d\n", adcValue);
return 0;
}
```
第二个程序:
```c
#include <stdio.h>
int main() {
int adcValue;
// 假设ADC引脚为A1
// 初始化ADC模块
// ...
// 开始读取ADC值
adcValue = readADC(1); // 读取A1引脚的ADC值
printf("ADC值: %d\n", adcValue);
return 0;
}
```
第三个程序:
```c
#include <stdio.h>
int main() {
int adcValue;
// 假设ADC引脚为A2
// 初始化ADC模块
// ...
// 开始读取ADC值
adcValue = readADC(2); // 读取A2引脚的ADC值
printf("ADC值: %d\n", adcValue);
return 0;
}
```
以上代码示例中使用假设的ADC引脚(A0, A1, A2),在实际应用中需要根据硬件连接情况进行相应的修改。初始化和读取ADC值的详细代码实现取决于使用的硬件平台和具体的ADC模块。
### 回答3:
ADC是模数转换器(Analog-to-Digital Converter)的缩写,用于将模拟信号转换为数字信号。下面是用C语言编写的三个ADC读取程序。
程序1:基础ADC读取程序
#include <stdio.h>
#include <stdint.h>
#define ADC_CHANNEL 0 // ADC通道号
int main(void) {
uint16_t adc_value;
// 初始化ADC
// ...
// 选择ADC通道
// ...
// 开始转换
// ...
// 等待转换完成
// ...
// 读取转换结果
adc_value = /* 从寄存器中获取ADC值 */;
printf("ADC Value: %d\n", adc_value);
return 0;
}
程序2:连续读取ADC值
#include <stdio.h>
#include <stdint.h>
#define ADC_CHANNEL 0 // ADC通道号
int main(void) {
uint16_t adc_value;
// 初始化ADC
// ...
// 选择ADC通道
// ...
while (1) {
// 开始转换
// ...
// 等待转换完成
// ...
// 读取转换结果
adc_value = /* 从寄存器中获取ADC值 */;
printf("ADC Value: %d\n", adc_value);
}
return 0;
}
程序3:使用中断方式读取ADC值
#include <stdio.h>
#include <stdint.h>
#define ADC_CHANNEL 0 // ADC通道号
void adc_interrupt_handler(void) {
uint16_t adc_value;
// 等待转换完成中断
// ...
// 读取转换结果
adc_value = /* 从寄存器中获取ADC值 */;
printf("ADC Value: %d\n", adc_value);
}
int main(void) {
// 初始化ADC中断
// ...
// 选择ADC通道
// ...
// 启用ADC转换完成中断
// ...
while (1) {
// 主程序执行其他任务
// ...
}
return 0;
}
以上是三个简单的ADC读取程序示例,具体实现取决于所使用的ADC芯片和开发环境。在实际应用中,需要根据硬件设备和要求进行适当的配置和修改。
相关推荐
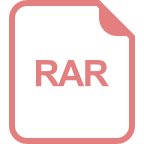
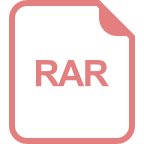














