帮我实现一个Linux下UDS转UART的C语言程序
时间: 2023-04-05 11:04:40 浏览: 171
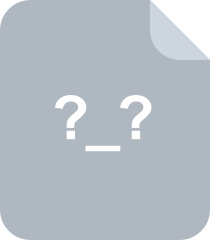
linux 下 UART 驱动程序

我可以回答这个问题。以下是一个简单的Linux下UDS转UART的C语言程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/socket.h>
#include <sys/un.h>
#include <fcntl.h>
#include <termios.h>
#define SOCK_PATH "/tmp/uds_socket"
#define UART_PATH "/dev/ttyS0"
int main(void)
{
int uds_sock, uart_fd, len;
struct sockaddr_un uds_addr;
struct termios uart_opts;
char buf[256];
// 创建UDS socket
if ((uds_sock = socket(AF_UNIX, SOCK_STREAM, 0)) == -1) {
perror("socket");
exit(1);
}
// 设置UDS地址
memset(&uds_addr, 0, sizeof(uds_addr));
uds_addr.sun_family = AF_UNIX;
strncpy(uds_addr.sun_path, SOCK_PATH, sizeof(uds_addr.sun_path) - 1);
// 连接UDS socket
if (connect(uds_sock, (struct sockaddr *)&uds_addr, sizeof(uds_addr)) == -1) {
perror("connect");
exit(1);
}
// 打开UART设备
if ((uart_fd = open(UART_PATH, O_RDWR | O_NOCTTY)) == -1) {
perror("open");
exit(1);
}
// 配置UART参数
memset(&uart_opts, 0, sizeof(uart_opts));
uart_opts.c_cflag = B9600 | CS8 | CLOCAL | CREAD;
uart_opts.c_iflag = IGNPAR;
uart_opts.c_oflag = 0;
uart_opts.c_lflag = 0;
tcflush(uart_fd, TCIFLUSH);
tcsetattr(uart_fd, TCSANOW, &uart_opts);
// 读取UDS socket数据并写入UART设备
while ((len = read(uds_sock, buf, sizeof(buf))) > 0) {
if (write(uart_fd, buf, len) != len) {
perror("write");
exit(1);
}
}
// 关闭UART设备和UDS socket
close(uart_fd);
close(uds_sock);
return 0;
}
```
这个程序创建了一个UDS socket,并连接到指定的地址。然后打开UART设备,并配置UART参数。最后,程序从UDS socket读取数据,并将其写入UART设备。
阅读全文
相关推荐
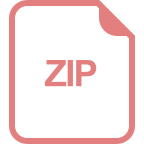
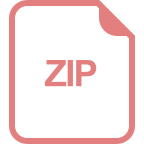
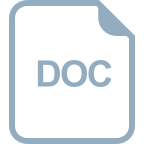
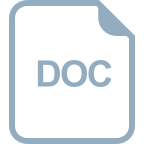












