C语言写一个带人机的五子棋游戏
时间: 2023-07-04 20:26:20 浏览: 81
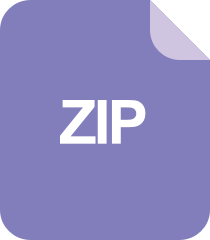
用C语言写一个五子棋游戏(人机)
好的,以下是一个简单的带人机的五子棋游戏的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define SIZE 15
int board[SIZE][SIZE];
void init_board() {
int i, j;
for (i = 0; i < SIZE; i++) {
for (j = 0; j < SIZE; j++) {
board[i][j] = 0;
}
}
}
void print_board() {
int i, j;
printf(" ");
for (i = 0; i < SIZE; i++) {
printf("%2d ", i + 1);
}
printf("\n");
for (i = 0; i < SIZE; i++) {
printf("%2d ", i + 1);
for (j = 0; j < SIZE; j++) {
if (board[i][j] == 0) {
printf(". ");
} else if (board[i][j] == 1) {
printf("O ");
} else if (board[i][j] == 2) {
printf("X ");
}
}
printf("\n");
}
}
int check_win(int player) {
int i, j, k;
for (i = 0; i < SIZE; i++) {
for (j = 0; j < SIZE; j++) {
if (board[i][j] == player) {
for (k = 1; k < 5; k++) {
if (i + k < SIZE && board[i + k][j] == player &&
i + k - 4 >= 0 && board[i + k - 1][j] == player &&
board[i + k - 2][j] == player && board[i + k - 3][j] == player) {
return 1;
}
if (j + k < SIZE && board[i][j + k] == player &&
j + k - 4 >= 0 && board[i][j + k - 1] == player &&
board[i][j + k - 2] == player && board[i][j + k - 3] == player) {
return 1;
}
if (i + k < SIZE && j + k < SIZE && board[i + k][j + k] == player &&
i + k - 4 >= 0 && j + k - 4 >= 0 && board[i + k - 1][j + k - 1] == player &&
board[i + k - 2][j + k - 2] == player && board[i + k - 3][j + k - 3] == player) {
return 1;
}
if (i + k < SIZE && j - k >= 0 && board[i + k][j - k] == player &&
i + k - 4 >= 0 && j - k + 4 < SIZE && board[i + k - 1][j - k + 1] == player &&
board[i + k - 2][j - k + 2] == player && board[i + k - 3][j - k + 3] == player) {
return 1;
}
}
}
}
}
return 0;
}
int player_move(int player) {
int row, col;
while (1) {
printf("Player %d's turn:\n", player);
printf("Enter row and column: ");
scanf("%d%d", &row, &col);
if (row >= 1 && row <= SIZE && col >= 1 && col <= SIZE && board[row - 1][col - 1] == 0) {
board[row - 1][col - 1] = player;
return 1;
} else {
printf("Invalid move! Please try again.\n");
}
}
}
int computer_move(int player) {
int row, col;
printf("Computer's turn:\n");
while (1) {
row = rand() % SIZE;
col = rand() % SIZE;
if (board[row][col] == 0) {
board[row][col] = player;
return 1;
}
}
}
int main() {
int player = 1;
int win = 0;
init_board();
srand(time(NULL));
while (!win) {
print_board();
if (player == 1) {
player_move(player);
} else {
computer_move(player);
}
win = check_win(player);
if (win) {
print_board();
printf("Player %d wins!\n", player);
} else {
player = player == 1 ? 2 : 1;
}
}
return 0;
}
```
该程序使用了一个二维数组来表示棋盘,其中0表示空位,1表示玩家1的棋子,2表示玩家2(即计算机)的棋子。程序中定义了两个函数,`player_move`和`computer_move`,分别表示玩家和计算机的走棋行为。其中,计算机的走棋行为是随机的。程序通过调用`check_win`函数来判断当前局面是否出现胜者,如果有则游戏结束。程序使用了随机数生成函数`srand`和`rand`,需要包含头文件`stdlib.h`和`time.h`。
阅读全文
相关推荐
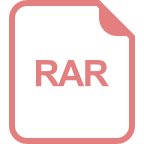















