使用c递归实现1-50中的偶数和
时间: 2024-02-27 09:58:12 浏览: 27
好的,以下是使用C语言递归实现1-50中的偶数和的代码:
```c
#include <stdio.h>
int sumEven(int n) {
if (n == 0) { // 递归终止条件
return 0;
}
if (n % 2 == 0) { // 如果是偶数
return n + sumEven(n-2); // 递归求解
}
else { // 如果是奇数
return sumEven(n-1); // 递归求解
}
}
int main() {
int sum = sumEven(50);
printf("1-50中的偶数和为:%d\n", sum);
return 0;
}
```
代码的思路是:如果n是偶数,则将n加入结果中,并递归调用sumEven(n-2);如果n是奇数,则递归调用sumEven(n-1)。当n等于0时,递归结束,返回0。最终得到1-50中的偶数和。
相关问题
c代码使用递归函数输出1-100的所有偶数
好的,以下是递归函数输出1~100的所有偶数的 C 代码实现:
```c
#include <stdio.h>
void print_even(int num) {
if (num > 100) { // 递归终止条件
return;
}
if (num % 2 == 0) { // 如果是偶数,输出
printf("%d\n", num);
}
print_even(num + 1); // 递归调用,继续判断下一个数
}
int main() {
print_even(1); // 调用函数开始输出偶数
return 0;
}
```
这个递归函数的思路与前面的 Python 版本类似:先判断当前数字是否大于 100,如果是则结束递归。若不是,则判断当前数字是否为偶数,如果是则输出,否则继续递归调用函数,判断下一个数字。这样就可以输出 1~100 的所有偶数了。
c语言递归实现指数函数
下面是使用递归实现指数函数的C语言代码:
```c
#include <stdio.h>
double power(double x, int n){
if(n == 0){
return 1;
}
if(n < 0){
return 1 / power(x, -n);
}
double half = power(x, n / 2);
if(n % 2 == 0){
return half * half;
}else{
return half * half * x;
}
}
int main(){
double x = 2.0;
int n = 5;
double result = power(x, n);
printf("%lf to the power of %d is: %lf\n", x, n, result);
return 0;
}
```
在这个代码中,我们定义了一个函数 `power`,它接受两个参数:一个是底数 `x`,另一个是指数 `n`。
在函数中,我们首先判断 `n` 是否等于0,如果是,返回1。然后判断 `n` 是否小于0,如果是,返回1除以 `x` 的 `-n` 次方。接下来,我们使用递归调用 `power` 函数来计算 `x` 的 `n/2` 次方,将结果存储在变量 `half` 中。如果 `n` 是偶数,我们返回 `half` 的平方;否则,我们返回 `half` 的平方乘以 `x`。
在 `main` 函数中,我们定义了底数和指数,并调用 `power` 函数进行计算。最后,我们输出计算结果。
相关推荐
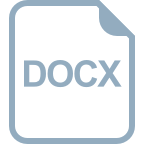
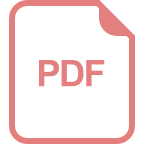
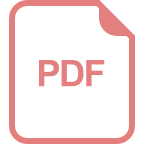












