帮我使用C++写一份公司管理系统,需要复杂一点,再加上注释
时间: 2024-03-03 20:49:51 浏览: 60
好的,我可以帮你写一份公司管理系统。由于公司管理系统的功能比较复杂,我会尽可能地详细解释每个部分的实现方式和注释。下面是一份基于C++的公司管理系统:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// Employee类表示公司员工
class Employee {
public:
Employee(string name, int age, string position, int salary) {
this->name = name;
this->age = age;
this->position = position;
this->salary = salary;
}
string getName() const { return name; }
int getAge() const { return age; }
string getPosition() const { return position; }
int getSalary() const { return salary; }
void setName(string name) { this->name = name; }
void setAge(int age) { this->age = age; }
void setPosition(string position) { this->position = position; }
void setSalary(int salary) { this->salary = salary; }
// 显示员工信息
void displayEmployee() const {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Position: " << position << endl;
cout << "Salary: " << salary << endl;
}
private:
string name;
int age;
string position;
int salary;
};
// Company类表示公司
class Company {
public:
// 添加员工
void addEmployee() {
string name, position;
int age, salary;
cout << "Enter employee name: ";
cin >> name;
cout << "Enter employee age: ";
cin >> age;
cout << "Enter employee position: ";
cin >> position;
cout << "Enter employee salary: ";
cin >> salary;
Employee employee(name, age, position, salary);
employees.push_back(employee);
cout << "Employee added successfully!" << endl;
}
// 显示所有员工信息
void displayAllEmployees() const {
for (const auto& employee : employees) {
employee.displayEmployee();
cout << endl;
}
}
// 显示员工薪资排名
void displaySalaryRanking() const {
vector<Employee> tempEmployees = employees;
sort(tempEmployees.begin(), tempEmployees.end(), [](const Employee& employee1, const Employee& employee2) {
return employee1.getSalary() > employee2.getSalary();
});
cout << "Salary Ranking:" << endl;
int rank = 1;
for (const auto& employee : tempEmployees) {
cout << rank << ". " << employee.getName() << " (" << employee.getSalary() << ")" << endl;
rank++;
}
}
// 更新员工信息
void updateEmployeeInfo() {
string name;
cout << "Enter employee name: ";
cin >> name;
bool found = false;
for (auto& employee : employees) {
if (employee.getName() == name) {
found = true;
string position;
int age, salary;
cout << "Enter new position: ";
cin >> position;
cout << "Enter new age: ";
cin >> age;
cout << "Enter new salary: ";
cin >> salary;
employee.setPosition(position);
employee.setAge(age);
employee.setSalary(salary);
cout << "Employee information updated successfully!" << endl;
break;
}
}
if (!found) {
cout << "Employee not found!" << endl;
}
}
// 删除员工
void deleteEmployee() {
string name;
cout << "Enter employee name: ";
cin >> name;
bool found = false;
for (auto it = employees.begin(); it != employees.end(); ++it) {
if (it->getName() == name) {
found = true;
employees.erase(it);
cout << "Employee deleted successfully!" << endl;
break;
}
}
if (!found) {
cout << "Employee not found!" << endl;
}
}
private:
vector<Employee> employees;
};
// 主函数
int main() {
Company company;
int choice;
while (true) {
cout << "1. Add employee" << endl;
cout << "2. Display all employees" << endl;
cout << "3. Display salary ranking" << endl;
cout << "4. Update employee information" << endl;
cout << "5. Delete employee" << endl;
cout << "6. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
company.addEmployee();
break;
case 2:
company.displayAllEmployees();
break;
case 3:
company.displaySalaryRanking();
break;
case 4:
company.updateEmployeeInfo();
break;
case 5:
company.deleteEmployee();
break;
case 6:
cout << "Goodbye!" << endl;
return 0;
default:
cout << "Invalid choice!" << endl;
break;
}
}
return 0;
}
```
在上面的代码中,我们定义了一个`Employee`类来表示公司员工,每个员工有名字、年龄、职位和薪水四个属性。`Company`类表示整个公司,其中包含了添加员工、显示所有员工信息、显示员工薪资排名、更新员工信息和删除员工等五个功能。
在主函数中,我们使用一个循环来实现菜单选择功能。用户可以通过输入不同的数字来选择不同的功能,然后调用相应的`Company`类的函数来实现相应的功能。
注释已经添加到代码中,希望对你有所帮助!
阅读全文
相关推荐











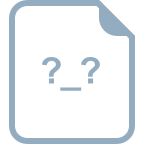
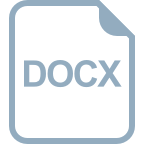