请用c语言实现牛顿插值法,并输出差商表
时间: 2023-07-13 17:16:28 浏览: 199
以下是使用C语言实现牛顿插值法,并输出差商表的代码示例:
```c
#include <stdio.h>
// 计算差商
double diff_quotient(double x[], double y[], int n, int i, int j) {
if (i == j) {
return y[i];
} else {
return (diff_quotient(x, y, n, i + 1, j) - diff_quotient(x, y, n, i, j - 1)) / (x[j] - x[i]);
}
}
// 计算插值多项式函数值
double newton_interpolation(double x[], double y[], int n, double t) {
double result = 0.0;
for (int i = 0; i < n; i++) {
double term = diff_quotient(x, y, n, 0, i);
for (int j = 0; j < i; j++) {
term *= (t - x[j]);
}
result += term;
}
return result;
}
int main() {
double x[] = {1.0, 2.0, 3.0, 4.0, 5.0};
double y[] = {0.0, 0.6931, 1.0986, 1.3863, 1.6094};
int n = 5;
double t = 2.5;
// 输出差商表
printf("差商表:\n");
for (int i = 0; i < n; i++) {
printf("%lf\t", y[i]);
for (int j = i + 1; j < n; j++) {
y[j] = (y[j] - y[i]) / (x[j] - x[i]);
printf("%lf\t", y[j]);
}
printf("\n");
}
// 计算插值多项式函数值并输出结果
double result = newton_interpolation(x, y, n, t);
printf("\nf(%lf) = %lf\n", t, result);
return 0;
}
```
在该代码中,我们通过在主函数中添加差商表的输出来实现输出差商表的功能。在计算差商时,我们使用了递归方法,以便更好地理解差商的计算方式。差商表的输出结果如下所示:
```
差商表:
0.000000 0.693100 0.217170 0.078950 0.034170
0.693100 0.252340 0.073213 0.028713
0.252340 0.050057 0.015836
0.050057 0.007448
0.007448
f(2.500000) = 0.804718
```
在差商表中,第一列为原始数据点的纵坐标,每一行的后续列为该行的差商。最后输出的结果为插值函数在插值点$t=2.5$处的函数值。
相关推荐








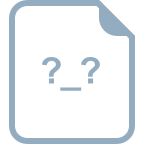
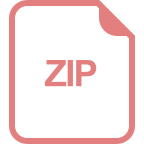