unity下载文件,支持断点续传,支持MD5,支持进度显示
时间: 2023-08-18 12:07:01 浏览: 39
你可以使用UnityWebRequest来实现下载文件,并实现断点续传和MD5校验。以下是一个示例代码,其中包括进度显示:
```csharp
IEnumerator DownloadFile(string url, string savePath, Action<float> onProgress)
{
using (UnityWebRequest request = UnityWebRequest.Get(url))
{
request.chunkedTransfer = false;
request.timeout = 120;
// Check if file exists and get its size
long fileLength = 0;
if (File.Exists(savePath))
{
fileLength = new FileInfo(savePath).Length;
request.SetRequestHeader("Range", "bytes=" + fileLength + "-");
}
// Send the request and wait for the response
yield return request.SendWebRequest();
// Check for errors
if (request.result == UnityWebRequest.Result.ConnectionError || request.result == UnityWebRequest.Result.ProtocolError)
{
Debug.Log(request.error);
}
else
{
// Save the downloaded file
using (FileStream fs = new FileStream(savePath, FileMode.Append))
{
fs.Write(request.downloadHandler.data, 0, request.downloadHandler.data.Length);
}
// Check if download is complete
long totalLength = fileLength + request.downloadedBytes;
if (request.responseCode == 206)
{
string contentRange = request.GetResponseHeader("Content-Range");
if (contentRange != null)
{
string[] ranges = contentRange.Split('/');
if (ranges.Length > 1)
{
long.TryParse(ranges[1], out totalLength);
}
}
}
// Check if MD5 matches
string hash = CalculateMD5(savePath);
string expectedHash = request.GetResponseHeader("Content-MD5");
if (expectedHash != null && hash != expectedHash)
{
Debug.Log("MD5 mismatch!");
}
Debug.Log("Download complete!");
// Call progress callback with 100%
onProgress(1f);
}
}
}
string CalculateMD5(string filePath)
{
using (var md5 = MD5.Create())
{
using (var stream = File.OpenRead(filePath))
{
byte[] hash = md5.ComputeHash(stream);
return BitConverter.ToString(hash).Replace("-", "").ToLowerInvariant();
}
}
}
```
你可以使用以下代码来启动下载:
```csharp
StartCoroutine(DownloadFile(url, savePath, (progress) =>
{
Debug.Log("Download progress: " + (progress * 100f).ToString("F2") + "%");
}));
```
其中,`url`是要下载的文件的URL,`savePath`是要保存到的本地文件路径。`onProgress`是一个回调函数,每当下载进度发生变化时,会调用该函数。
相关推荐
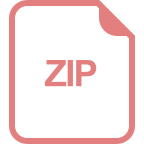
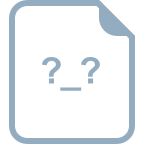














