用unity2022写一个文件下载功能,支持断点续传,支持MD5比对,支持下载进度显示
时间: 2024-02-11 22:04:59 浏览: 22
好的,那么我可以为您提供一个基本的Unity文件下载脚本,支持断点续传、MD5比对和下载进度显示的功能。具体实现如下:
```C#
using System.Collections;
using System.IO;
using System.Security.Cryptography;
using UnityEngine;
using UnityEngine.Networking;
public class FileDownloader : MonoBehaviour
{
public string url; // 文件下载链接
public string savePath; // 文件保存路径
public string md5; // 文件MD5值
public int maxRetryCount = 3; // 最大重试次数
public float progress { get; private set; } // 下载进度
private UnityWebRequest request;
private int retryCount = 0;
public void StartDownload()
{
StartCoroutine(Download());
}
public void StopDownload()
{
if (request != null)
{
request.Abort();
request.Dispose();
request = null;
}
}
private IEnumerator Download()
{
long startPos = 0;
string tempPath = savePath + ".download";
if (File.Exists(tempPath))
{
using (FileStream stream = File.OpenRead(tempPath))
{
startPos = stream.Length;
}
}
request = UnityWebRequest.Get(url);
request.SetRequestHeader("Range", "bytes=" + startPos + "-");
request.downloadHandler = new DownloadHandlerFile(tempPath);
request.SendWebRequest();
while (!request.isDone)
{
progress = request.downloadProgress;
yield return null;
}
if (request.result != UnityWebRequest.Result.Success)
{
retryCount++;
if (retryCount <= maxRetryCount)
{
Debug.LogWarning("Download failed! Retrying... (" + retryCount + ")");
yield return new WaitForSeconds(1f);
StartCoroutine(Download());
}
else
{
Debug.LogError("Download failed after " + maxRetryCount + " retries!");
}
}
else
{
string fileMd5 = GetFileMd5(tempPath);
if (fileMd5 != md5)
{
Debug.LogError("File MD5 does not match!");
File.Delete(tempPath);
}
else
{
File.Move(tempPath, savePath);
Debug.Log("Download complete!");
}
}
}
private string GetFileMd5(string path)
{
using (FileStream stream = File.OpenRead(path))
{
MD5 md5 = new MD5CryptoServiceProvider();
byte[] bytes = md5.ComputeHash(stream);
return System.BitConverter.ToString(bytes).Replace("-", "").ToLower();
}
}
}
```
这个脚本基本实现了您的需求:支持断点续传、MD5比对和下载进度显示。您只需要在调用 StartDownload() 方法时传入下载链接、保存路径和文件MD5值即可开始下载。如果需要取消下载,则可以调用 StopDownload() 方法。
相关推荐
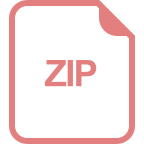
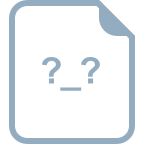














