unity2021下载文件,支持断点续传,支持MD5,支持进度条显示 webRequest.result != UnityWebRequest.Result.Success
时间: 2024-02-11 07:03:37 浏览: 31
你可以使用UnityWebRequest来实现这个功能。UnityWebRequest支持断点续传、MD5校验和进度条显示,并且可以通过检查webRequest.result属性来判断请求是否成功。
以下是一个示例代码,演示如何下载文件并显示下载进度:
```csharp
using System.Collections;
using UnityEngine;
using UnityEngine.Networking;
public class FileDownloader : MonoBehaviour
{
public string fileUrl;
public string savePath;
private UnityWebRequest webRequest;
public IEnumerator DownloadFile()
{
webRequest = UnityWebRequest.Get(fileUrl);
webRequest.downloadHandler = new DownloadHandlerFile(savePath);
// 获取已下载的字节数
long startPos = GetExistingFileSize(savePath);
if (startPos > 0)
{
// 设置断点续传的起始位置
webRequest.SetRequestHeader("Range", "bytes=" + startPos + "-");
}
webRequest.SendWebRequest();
while (!webRequest.isDone)
{
float progress = webRequest.downloadProgress;
Debug.Log("Download progress: " + progress);
yield return null;
}
if (webRequest.result == UnityWebRequest.Result.ConnectionError ||
webRequest.result == UnityWebRequest.Result.DataProcessingError ||
webRequest.result == UnityWebRequest.Result.ProtocolError)
{
Debug.LogError("Download failed: " + webRequest.error);
}
else
{
Debug.Log("Download complete!");
// 验证文件MD5
string md5 = ComputeMD5(savePath);
if (md5 != GetFileMD5(fileUrl))
{
Debug.LogError("MD5 check failed!");
}
}
}
// 获取已下载的字节数
private long GetExistingFileSize(string filePath)
{
if (File.Exists(filePath))
{
FileInfo fileInfo = new FileInfo(filePath);
return fileInfo.Length;
}
return 0;
}
// 计算文件的MD5值
private string ComputeMD5(string filePath)
{
using (var md5 = System.Security.Cryptography.MD5.Create())
{
using (var stream = File.OpenRead(filePath))
{
byte[] hash = md5.ComputeHash(stream);
return BitConverter.ToString(hash).Replace("-", "").ToLower();
}
}
}
// 获取文件的MD5值
private string GetFileMD5(string fileUrl)
{
using (var webClient = new WebClient())
{
byte[] data = webClient.DownloadData(fileUrl);
using (var md5 = System.Security.Cryptography.MD5.Create())
{
byte[] hash = md5.ComputeHash(data);
return BitConverter.ToString(hash).Replace("-", "").ToLower();
}
}
}
}
```
在上面的示例中,我们通过UnityWebRequest.Get方法创建一个下载请求,设置下载进度和下载完成后保存文件的路径。我们使用SetRequestHeader方法设置断点续传的起始位置,使用ComputeMD5方法计算下载完成后文件的MD5值,并使用GetFileMD5方法获取文件在服务器上的MD5值,最后对比两个MD5值是否相同。
注意:在下载文件之前,需要确保设置了网络权限。在Android平台上,需要在AndroidManifest.xml文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
这样就可以通过UnityWebRequest来下载文件,并支持断点续传、MD5校验和进度条显示了。
相关推荐
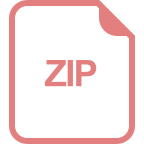
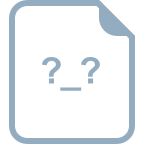














