帮我用unity写一个文件下载器,下载未完成时存储为临时文件,当文件下载完成则转为正式文件,支持断点续传,显示下载进度
时间: 2024-01-08 19:04:54 浏览: 22
好的,我可以提供一个简单的文件下载器的实现代码,你可以参考一下。
```csharp
using System.Collections;
using System.IO;
using UnityEngine;
using UnityEngine.Networking;
using UnityEngine.UI;
public class FileDownloader : MonoBehaviour
{
public string downloadUrl;
public string savePath;
public Text progressText;
private UnityWebRequest request;
private string tempFilePath;
private long downloadedBytes;
void Start()
{
// 创建临时文件路径
tempFilePath = savePath + ".download";
// 检查是否存在临时文件,如果存在,则尝试断点续传
if (File.Exists(tempFilePath))
{
downloadedBytes = new FileInfo(tempFilePath).Length;
request = UnityWebRequest.Get(downloadUrl);
request.SetRequestHeader("Range", "bytes=" + downloadedBytes + "-");
}
else
{
request = UnityWebRequest.Get(downloadUrl);
}
StartCoroutine(DownloadFile());
}
IEnumerator DownloadFile()
{
yield return request.SendWebRequest();
if (request.result == UnityWebRequest.Result.ConnectionError || request.result == UnityWebRequest.Result.ProtocolError)
{
Debug.LogError(request.error);
}
else
{
// 下载完成,将临时文件重命名为正式文件
File.Move(tempFilePath, savePath);
Debug.Log("Download complete!");
}
}
void Update()
{
if (request.isDone)
{
progressText.text = "100%";
}
else
{
// 更新下载进度
float progress = (float)(downloadedBytes + request.downloadedBytes) / request.downloadedBytes;
progressText.text = string.Format("{0:F2}%", progress * 100);
}
// 检查是否需要保存临时文件
if (request.downloadedBytes > downloadedBytes)
{
downloadedBytes = request.downloadedBytes;
using (FileStream fs = new FileStream(tempFilePath, FileMode.Append))
{
byte[] buffer = request.downloadHandler.data;
fs.Write(buffer, 0, buffer.Length);
}
}
}
}
```
这个脚本中包含了一个 `Start()` 方法和一个协程 `DownloadFile()`。在 `Start()` 方法中,我们首先检查是否存在临时文件,如果存在则尝试断点续传,否则直接启动下载。在协程 `DownloadFile()` 中,我们使用 `UnityWebRequest` 类来进行文件下载,并在下载过程中保存已经下载的数据到临时文件中。当整个文件下载完成后,将临时文件重命名为正式文件。
在 `Update()` 方法中,我们更新下载进度,并且检查是否需要保存临时文件。注意,我们在保存临时文件时使用了 `FileStream.Append` 模式,以便将新下载的数据追加到已有的数据之后。
另外,这个脚本还包含了一个 `progressText` 公开变量,用于在 UI 中显示下载进度。
这只是一个简单的实现,如果你需要更复杂的功能(比如支持多线程下载、支持下载队列等),你需要进行更多的工作。希望这个实现能对你有所启发。
相关推荐
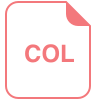
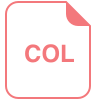
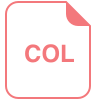
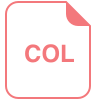
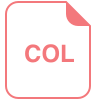









